Introduction
Ever needed to take away an merchandise from an inventory however not simply any merchandise, particularly the one at a sure index? Enter Python pop() technique. This built-in operate helps you obtain precisely that. It removes a component from an inventory primarily based on its index and, most significantly, returns the eliminated ingredient, supplying you with management over your knowledge buildings. Whether or not you’re managing dynamic lists, dealing with consumer enter, or manipulating arrays, understanding python pop() can prevent effort and time. Let’s discover this useful instrument in depth.
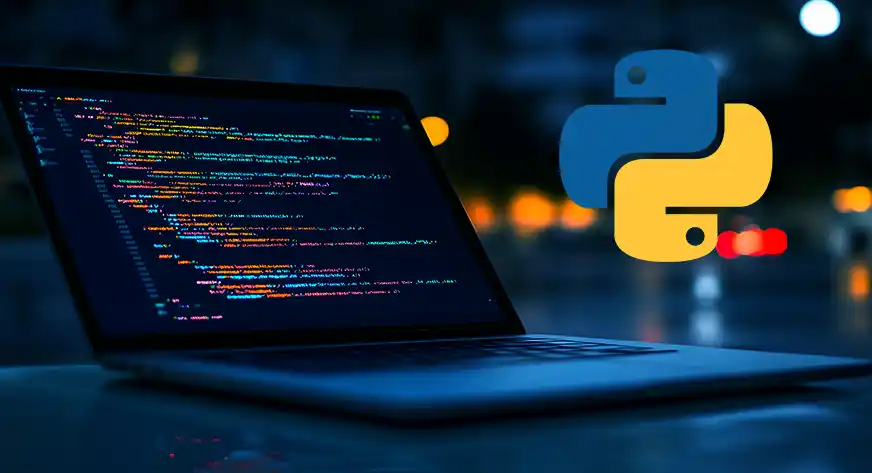
Studying Outcomes
- Perceive the aim and syntax of the
pop()
technique in Python. - Learn to take away parts from an inventory utilizing
pop()
. - Know how one can work with the index parameter in
pop()
to take away particular parts. - Perceive error dealing with when
pop()
is used incorrectly. - See sensible examples of utilizing
pop()
in varied eventualities.
What’s the pop()
Technique in Python?
The pop()
technique in Python is used to take away an merchandise from a record at a specified place (index). It additionally returns the worth of the eliminated ingredient. In contrast to strategies like take away()
, which require the worth of the ingredient, pop()
works with indices, supplying you with extra management over which merchandise you wish to remove from the record.
Syntax:
record.pop(index)
- record: The record you wish to take away a component from.
- index (optionally available): The index of the ingredient to take away. If no index is offered,
pop()
will take away the final ingredient by default.
How Does the pop()
Technique Work?
The pop()
technique in Python works by immediately altering the record in place and returning the ingredient it removes. This technique supplies flexibility in eradicating parts both by specifying their place within the record or by defaulting to the final ingredient. Under are detailed explanations of how pop()
behaves in varied eventualities:
Index Specified
In case you use the pop() technique and specify an index, this operate deletes a component by this index and brings the worth of the deleted ingredient. The record could be additionally modified in place, that signifies that the unique record is altering its content material.
The way it works?
- The ingredient to be deleted is searched utilizing the assistance of the required index.
- After the ingredient is discovered, it’s deleted and all of the others to the proper of the index are moved one step to the left to take the place of the deleted ingredient.
- The eliminated ingredient is returned: it may be saved in a variable or used with out being assigned to one thing.
Instance:
my_list = ['apple', 'banana', 'cherry', 'date']
removed_element = my_list.pop(1) # Removes ingredient at index 1, which is 'banana'
print(removed_element) # Output: 'banana'
print(my_list) # Output: ['apple', 'cherry', 'date']
Index Not Specified
If no index is handed to operate pop(), then it removes the final merchandise of the record. That is useful when it’s worthwhile to delete the most recent or the final ingredient with out the necessity for figuring the index.
The way it works?
- For example, if no index has been outlined, then pop() immediately refers back to the final merchandise within the record, the identical with (-1).
- The ultimate worth within the record is then taken away and the strategy then returns the worth related to such parameter.
Instance:
my_list = [10, 20, 30, 40]
removed_element = my_list.pop() # Removes the final ingredient, 40
print(removed_element) # Output: 40
print(my_list) # Output: [10, 20, 30]
IndexError
Python raises an IndexError
should you try to make use of the pop()
technique on an empty record or present an invalid index that’s out of bounds. This habits ensures that the record can’t be accessed or modified past its accessible vary.
- Popping from an Empty Checklist: In case you attempt to pop a component from an empty record, Python raises an
IndexError
as a result of there aren’t any parts to take away. This prevents undefined habits and alerts you that the operation is invalid.
Instance:
empty_list = []
empty_list.pop() # Raises IndexError: pop from empty record
- Invalid Index: In case you present an index that’s higher than or equal to the size of the record, Python raises an
IndexError
as a result of that index doesn’t exist inside the record’s bounds.
Instance:
my_list = [1, 2, 3]
my_list.pop(10) # Raises IndexError: pop index out of vary
Damaging Indexing Utilizing pop() Operate
Lists in Python have the aptitude of adverse indexing, this simply signifies that you’ll be able to depend from the final index backwards. One other technique that helps that is the pop() technique. A adverse index can be utilized to cross to the pop() operate with the intention to delete parts from the record by the top.
Instance:
my_list = [100, 200, 300, 400]
removed_item = my_list.pop(-2) # Removes the second-to-last ingredient
print(removed_item) # Output: 300
print(my_list) # Output: [100, 200, 400]
Utilizing pop()
with Dictionaries in Python
The pop() technique works not solely with record sort objects in Python, however with dictionaries as effectively. Based mostly on that, the pop() technique is utility to dictionaries the place a key and faraway from the record and the worth akin to that key’s returned.
That is particularly necessary if it’s important to use the ‘pop’ technique to get and delete a component from the dictionary, on the similar time.
When performing pop() on the dictionary, you’ll cross the important thing within the dictionary whose worth you want to receive and delete directly. If the bottom line is current in dictionary then the pop() features will return the worth and in addition eliminates the key-value pair from the dictionary. If the important thing doesn’t exist, you possibly can both make Python throw the KeyError for you or provide a second worth that the operate ought to use if the important thing doesn’t exist within the dictionary.
Examples of Utilizing pop()
with Dictionaries
Allow us to discover totally different examples of utilizing pop() with dictionaries:
Primary Utilization
scholar = {'title': 'John', 'age': 25, 'course': 'Arithmetic'}
age = scholar.pop('age')
print(age) # Output: 25
print(scholar) # Output: {'title': 'John', 'course': 'Arithmetic'}
Offering a Default Worth
If the important thing doesn’t exist within the dictionary, you possibly can forestall the KeyError
by offering a default worth.
scholar = {'title': 'John', 'age': 25, 'course': 'Arithmetic'}
main = scholar.pop('main', 'Not discovered')
print(main) # Output: Not discovered
print(scholar) # Output: {'title': 'John', 'age': 25, 'course': 'Arithmetic'}
Dealing with KeyError
With no Default Worth
In case you don’t present a default worth and the bottom line is lacking, Python will elevate a KeyError
.
scholar = {'title': 'John', 'age': 25, 'course': 'Arithmetic'}
grade = scholar.pop('grade') # KeyError: 'grade'
How pop()
Impacts Reminiscence
If an inventory is resized and a component is faraway from the record utilizing the pop technique then it alters the pointer of the record. The lists in Python are dynamic arrays, so manipulating on them resembling append or pop will truly change the reminiscence allocation of the record.
Right here’s an in depth clarification of how pop()
impacts reminiscence:
Dynamic Array and Reminiscence Administration
Python lists are dynamic arrays, that means they’re saved in contiguous blocks of reminiscence. While you take away a component utilizing pop()
, Python should reallocate or shift parts to take care of this contiguous reminiscence construction.
- If the ingredient eliminated is not the final one (i.e., you present a particular index), Python shifts all the weather that come after the eliminated ingredient to fill the hole.
- In case you take away the final ingredient (i.e., no index is offered), there isn’t any want for shifting; Python merely updates the record’s inner dimension reference.
Inner Reminiscence Allocation
Lists in Python are over-allocated, that means they reserve further house to accommodate future parts with out requiring speedy reallocation of reminiscence. This minimizes the overhead of steadily resizing the record when parts are added or eliminated. Nonetheless, eradicating parts with pop()
decreases the scale of the record, and over time, Python may determine to launch unused reminiscence, though this doesn’t occur instantly after a single pop()
operation.
Shifting Parts in Reminiscence
While you take away a component from the center of an inventory, the opposite parts within the record shift to the left to fill the hole. This course of entails Python shifting knowledge round in reminiscence, which could be time-consuming, particularly if the record is giant. Since Python has to bodily shift every ingredient one step over, this could develop into inefficient because the record grows in dimension.
Instance:
my_list = [10, 20, 30, 40, 50]
my_list.pop(1) # Removes the ingredient at index 1, which is 20
print(my_list) # Output: [10, 30, 40, 50]
Reminiscence Effectivity for Massive Lists
When working with giant lists, frequent use of pop()
(particularly from indices apart from the final one) may cause efficiency degradation due to the repeated must shift parts. Nonetheless, eradicating parts from the top of the record (utilizing pop()
with no index) is environment friendly and doesn’t contain shifting.
Effectivity of pop()
The effectivity of the pop()
technique will depend on the place within the record the ingredient is being eliminated. Under are particulars on the time complexity for various eventualities:
Greatest Case (O(1) – Eradicating the Final Aspect)
- When no index is specified,
pop()
removes the final ingredient within the record. This operation is fixed time, O(1), as a result of no different parts should be shifted. - Eradicating the final ingredient solely entails reducing the record’s dimension by one and doesn’t require modifying the positions of some other parts in reminiscence.
Instance:
my_list = [5, 10, 15, 20]
my_list.pop() # Removes the final ingredient, 20
print(my_list) # Output: [5, 10, 15]
Worst Case (O(n) – Eradicating the First Aspect)
- When an index is specified (significantly when it’s 0, the primary ingredient),
pop()
turns into a linear time operation, O(n), as a result of each ingredient after the eliminated one have to be shifted one place to the left. - The bigger the record, the extra parts should be shifted, making this much less environment friendly for big lists.
Instance:
my_list = [1, 2, 3, 4, 5]
my_list.pop(0) # Removes the primary ingredient, 1
print(my_list) # Output: [2, 3, 4, 5]
Intermediate Circumstances (O(n) – Eradicating from the Center)
Even, should you take an merchandise out at a few of random place like pop(5), it additionally takes solely O(n) time the place n is the variety of parts after the fifth that they’re compelled to shift. The nearer the worth of the index is to the utmost representing the final ingredient within the record, the much less operations that must be carried out to execute it making it much less environment friendly than the straight pop however extra environment friendly if one compares it with a pop entrance.
Instance:
my_list = [10, 20, 30, 40, 50]
my_list.pop(2) # Removes the ingredient at index 2, which is 30
print(my_list) # Output: [10, 20, 40, 50]
Comparability with take away()
The pop()
and take away()
strategies each delete parts from an inventory, however they behave in a different way and serve distinct functions. Right here’s an in depth comparability between the 2:
Function | pop() Technique |
take away() Technique |
---|---|---|
What It Does | Takes out an merchandise from the record and offers it again to you. You’ll be able to both specify which place (index) or depart it empty to take away the final merchandise. | Appears to be like for a particular worth within the record and removes it. No return worth, simply deletes it. |
How It Returns Values | Provides you the eliminated merchandise. | Doesn’t return something – it simply deletes the merchandise. |
Utilizing an Index | Works with indices, letting you decide an merchandise primarily based on its place. | Doesn’t help indices – solely removes primarily based on worth. |
Utilizing a Worth | You’ll be able to’t take away an merchandise by its worth, solely by its place. | You’ll be able to take away an merchandise by immediately specifying the worth. |
Errors You Would possibly Encounter | If the index is flawed or the record is empty, it throws an IndexError . |
If the worth isn’t within the record, you’ll get a ValueError . |
When to Use | Nice if you want each to take away an merchandise and use its worth afterward. | Greatest when the worth you wish to take away, however don’t must get it again. |
Conclusion
The pop() technique is without doubt one of the most helpful strategies of Python relating to erasing objects from an inventory. From dealing with record stacks, growing your record dynamism and even erasing the final merchandise utilizing pop(), all of it will get made simpler by understanding how one can use this function in Python. Watch out with IndexError whereas attempting to entry legitimate indices particularly whereas coping with empty lists.
Regularly Requested Questions
pop()
technique?
A. If no index is handed, pop()
removes and returns the final ingredient of the record by default.
pop()
on an empty record?
A. No, calling pop()
on an empty record will elevate an IndexError
. You need to make sure the record is just not empty earlier than utilizing pop()
.
pop()
deal with adverse indices?
A. pop()
can work with adverse indices. For instance, pop(-1)
removes the final ingredient, pop(-2)
removes the second-to-last ingredient, and so forth.
pop()
be used with strings or tuples?
A. No, pop()
is particular to lists. Strings and tuples are immutable, that means their parts can’t be modified or eliminated.
pop()
take away all occurrences of a component?
A. No, pop()
solely removes a single ingredient at a particular index, not all occurrences of that ingredient within the record. If it’s worthwhile to take away all occurrences, you should use a loop or record comprehension.