Dealing with lacking knowledge is among the commonest challenges in knowledge evaluation and machine studying. Lacking values can come up for varied causes, comparable to errors in knowledge assortment, guide omissions, and even the pure absence of knowledge. Whatever the trigger, these gaps can considerably impression your evaluation’s or predictive fashions’ high quality and accuracy.
Pandas, one of the in style Python libraries for knowledge manipulation, gives strong instruments to cope with lacking values successfully. Amongst these, the fillna() technique stands out as a flexible and environment friendly technique to deal with lacking knowledge by imputation. This technique lets you change lacking values with a particular worth, the imply, median, mode, and even forward- and backward-fill methods, making certain that your dataset is full and analysis-ready.
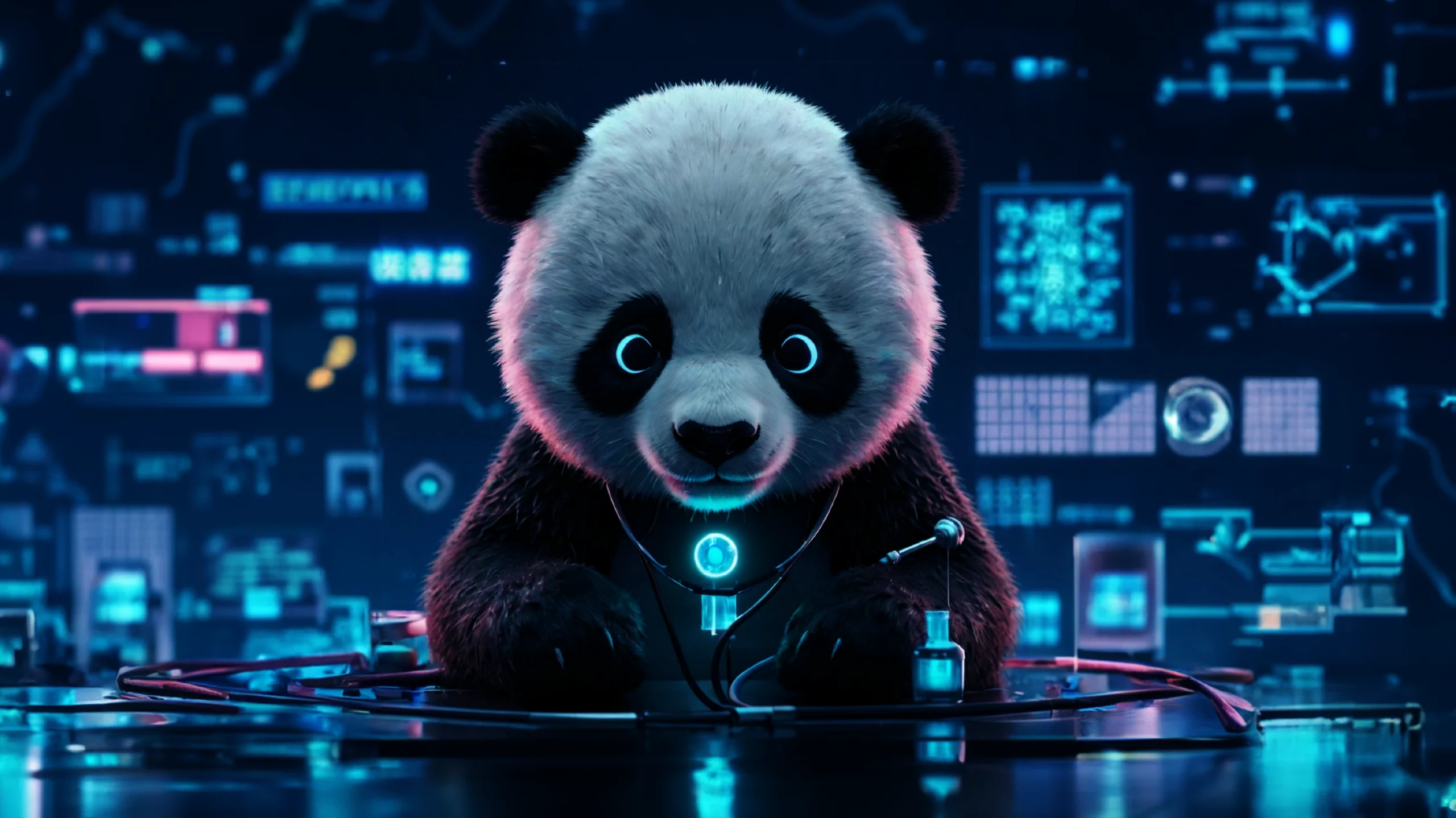
What’s Knowledge Imputation?
Knowledge imputation is the method of filling in lacking or incomplete knowledge in a dataset. When knowledge is lacking, it might probably create issues in evaluation, as many algorithms and statistical methods require an entire dataset to perform correctly. Knowledge imputation addresses this problem by estimating and changing the lacking values with believable ones, based mostly on the present knowledge within the dataset.
Why is Knowledge Imputation Vital?
Right here’s why:
Distorted Dataset
- Lacking knowledge can skew the distribution of variables, altering the dataset’s integrity. This distortion might result in anomalies, change the relative significance of classes, and produce deceptive outcomes.
- For instance, a excessive variety of lacking values in a selected demographic group might trigger incorrect weighting in a survey evaluation.
Limitations with Machine Studying Libraries
- Most machine studying libraries, comparable to Scikit-learn, assume that datasets are full. Lacking values could cause errors or forestall the profitable execution of algorithms, as these instruments typically lack built-in mechanisms for dealing with such points.
- Builders should preprocess the information to deal with lacking values earlier than feeding it into these fashions.
Impression on Mannequin Efficiency
- Lacking knowledge introduces bias, resulting in inaccurate predictions and unreliable insights. A mannequin skilled on incomplete or improperly dealt with knowledge would possibly fail to generalize successfully.
- As an illustration, if revenue knowledge is lacking predominantly for a particular group, the mannequin might fail to seize key traits associated to that group.
Need to Restore Dataset Completeness
- In instances the place knowledge is essential or datasets are small, dropping even a small portion can considerably impression the evaluation. Imputation turns into important to retain all obtainable data whereas mitigating the results of lacking knowledge.
- For instance, a small medical examine dataset would possibly lose statistical significance if rows with lacking values are eliminated.
Additionally learn: Pandas Features for Knowledge Evaluation and Manipulation
Understanding fillna() in Pandas
The fillna() technique replaces lacking values (NaN) in a DataFrame or Collection with specified values or computed ones. Lacking values can come up attributable to varied causes, comparable to incomplete knowledge entry or knowledge extraction errors. Addressing these lacking values ensures the integrity and reliability of your evaluation or mannequin.
Syntax of fillna() in Pandas
There are some necessary parameters obtainable in fillna():
- worth: Scalar, dictionary, Collection, or DataFrame to fill the lacking values.
- technique: Imputation technique. Might be:
- ‘ffill’ (ahead fill): Replaces NaN with the final legitimate worth alongside the axis.
- ‘bfill’ (backward fill): Replaces NaN with the following legitimate worth.
- axis: Axis alongside which to use the tactic (0 for rows, 1 for columns).
- inplace: If True, modifies the unique object.
- restrict: Most variety of consecutive NaNs to fill.
- downcast: Makes an attempt to downcast the ensuing knowledge to a smaller knowledge kind.
Utilizing fillna() for Totally different Knowledge Imputation Strategies
There are a number of knowledge Imputation methods which goals to protect the dataset’s construction and statistical properties whereas minimizing bias. These strategies vary from easy statistical approaches to superior machine learning-based methods, every suited to particular kinds of knowledge and missingness patterns.
We are going to see a few of these methods which will be applied with fillna():
1. Subsequent or Earlier Worth
For time-series or ordered knowledge, imputation strategies typically leverage the pure order of the dataset, assuming that close by values are extra comparable than distant ones. A typical method replaces lacking values with both the following or earlier worth within the sequence. This system works nicely for each nominal and numerical knowledge.
import pandas as pd
knowledge = {'Time': [1, 2, 3, 4, 5], 'Worth': [10, None, None, 25, 30]}
df = pd.DataFrame(knowledge)
# Ahead fill
df_ffill = df.fillna(technique='ffill')
# Backward fill
df_bfill = df.fillna(technique='bfill')
print(df_ffill)
print(df_bfill)
Additionally learn: Efficient Methods for Dealing with Lacking Values in Knowledge Evaluation
2. Most or Minimal Worth
When the information is understood to fall inside a particular vary, lacking values will be imputed utilizing both the utmost or minimal boundary of that vary. This technique is especially helpful when knowledge assortment devices saturate at a restrict. For instance, if a worth cap is reached in a monetary market, the lacking worth will be changed with the utmost allowable worth.
import pandas as pd
knowledge = {'Time': [1, 2, 3, 4, 5], 'Worth': [10, None, None, 25, 30]}
df = pd.DataFrame(knowledge)
# Impute lacking values with the minimal worth of the column
df_min = df.fillna(df.min())
# Impute lacking values with the utmost worth of the column
df_max = df.fillna(df.max())
print(df_min)
print(df_max)
3. Imply Imputation
Imply Imputation includes changing lacking values with the imply (common) worth of the obtainable knowledge within the column. This can be a easy method that works nicely when the information is comparatively symmetrical and freed from outliers. The imply represents the central tendency of the information, making it an affordable alternative for imputation when the dataset has a traditional distribution. Nevertheless, the key downside of utilizing the imply is that it’s delicate to outliers. Excessive values can skew the imply, resulting in an imputation that won’t replicate the true distribution of the information. Subsequently, it’s not ultimate for datasets with vital outliers or skewed distributions.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Imply Imputation
df['A_mean'] = df['A'].fillna(df['A'].imply())
print("Dataset after Imputation:")
print(df)
4. Median Imputation
Median Imputation replaces lacking values with the median worth, which is the center worth when the information is ordered. This technique is very helpful when the information comprises outliers or is skewed. In contrast to the imply, the median is not affected by excessive values, making it a extra strong alternative in such instances. When the information has a excessive variance or comprises outliers that might distort the imply, the median gives a greater measure of central tendency. Nevertheless, one draw back is that it might not seize the complete variability within the knowledge, particularly in datasets that comply with a regular distribution. Thus, in such instances, the imply would usually present a extra correct illustration of the information’s true central worth.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Median Imputation
df['A_median'] = df['A'].fillna(df['A'].median())
print("Dataset after Imputation:")
print(df)
5. Transferring Common Imputation
The Transferring Common Imputation technique calculates the common of a specified variety of surrounding values, referred to as a “window,” and makes use of this common to impute lacking knowledge. This technique is especially useful for time-series knowledge or datasets the place observations are associated to earlier or subsequent ones. The transferring common helps easy out fluctuations, offering a extra contextual estimate for lacking values. It’s generally used to deal with gaps in time-series knowledge, the place the belief is that close by values are prone to be extra comparable. The foremost drawback is that it might probably introduce bias if the information has giant gaps or irregular patterns, and it can be computationally intensive for giant datasets or advanced transferring averages. Nevertheless, it’s extremely efficient in capturing temporal relationships throughout the knowledge.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Transferring Common Imputation (utilizing a window of two)
df['A_moving_avg'] = df['A'].fillna(df['A'].rolling(window=2, min_periods=1).imply())
print("Dataset after Imputation:")
print(df)
6. Rounded Imply Imputation
The Rounded Imply Imputation method includes changing lacking values with the rounded imply worth. This technique is usually utilized when the information has a particular precision or scale requirement, comparable to when coping with discrete values or knowledge that must be rounded to a sure decimal place. As an illustration, if a dataset comprises values with two decimal locations, rounding the imply to 2 decimal locations ensures that the imputed values are per the remainder of the information. This method makes the information extra interpretable and aligns the imputation with the precision degree of the dataset. Nevertheless, a draw back is that rounding can result in a lack of precision, particularly in datasets the place fine-grained values are essential for evaluation.
import pandas as pd
import numpy as np
# Pattern dataset with lacking values
knowledge = {'A': [1, 2, np.nan, 4, 5, np.nan, 7],
'B': [10, np.nan, 30, 40, np.nan, 60, 70]}
df = pd.DataFrame(knowledge)
# Rounded Imply Imputation
df['A_rounded_mean'] = df['A'].fillna(spherical(df['A'].imply()))
print("Dataset after Imputation:")
print(df)
7. Fastened Worth Imputation
Fastened worth imputation is a straightforward and versatile method for dealing with lacking knowledge by changing lacking values with a predetermined worth, chosen based mostly on the context of the dataset. For categorical knowledge, this would possibly contain substituting lacking responses with placeholders like “not answered” or “unknown,” whereas numerical knowledge would possibly use 0 or one other fastened worth that’s logically significant. This method ensures consistency and is simple to implement, making it appropriate for fast preprocessing. Nevertheless, it might introduce bias if the fastened worth doesn’t replicate the information’s distribution, probably decreasing variability and impacting mannequin efficiency. To mitigate these points, it is very important select contextually significant values, doc the imputed values clearly, and analyze the extent of missingness to evaluate the imputation’s impression.
import pandas as pd
# Pattern dataset with lacking values
knowledge = {
'Age': [25, None, 30, None],
'Survey_Response': ['Yes', None, 'No', None]
}
df = pd.DataFrame(knowledge)
# Fastened worth imputation
# For numerical knowledge (e.g., Age), change lacking values with a hard and fast quantity, comparable to 0
df['Age'] = df['Age'].fillna(0)
# For categorical knowledge (e.g., Survey_Response), change lacking values with "Not Answered"
df['Survey_Response'] = df['Survey_Response'].fillna('Not Answered')
print("nDataFrame after Fastened Worth Imputation:")
print(df)
Additionally learn: An Correct Method to Knowledge Imputation
Conclusion
Dealing with lacking knowledge successfully is essential for sustaining the integrity of datasets and making certain the accuracy of analyses and machine studying fashions. Pandas fillna() technique provides a versatile and environment friendly method to knowledge imputation, accommodating a wide range of methods tailor-made to totally different knowledge varieties and contexts.
From easy strategies like changing lacking values with fastened values or statistical measures (imply, median, mode) to extra subtle methods like ahead/backward filling and transferring averages, every technique has its strengths and is suited to particular situations. By selecting the suitable imputation method, practitioners can mitigate the impression of lacking knowledge, reduce bias, and protect the dataset’s statistical properties.
In the end, choosing the appropriate imputation technique requires understanding the character of the dataset, the sample of missingness, and the targets of the evaluation. With instruments like fillna(), knowledge scientists and analysts are outfitted to deal with lacking knowledge effectively, enabling strong and dependable leads to their workflows.
In case you are on the lookout for an AI/ML course on-line, then, discover: Licensed AI & ML BlackBelt PlusProgram
Often Requested Questions
Ans. The fillna() technique in Pandas is used to interchange lacking values (NaN) in a DataFrame or Collection with a specified worth, technique, or computation. It permits filling with a hard and fast worth, propagating the earlier or subsequent legitimate worth utilizing strategies like ffill (ahead fill) or bfill (backward fill), or making use of totally different methods column-wise with dictionaries. This perform is crucial for dealing with lacking knowledge and making certain datasets are full for evaluation.
Ans. The first distinction between dropna() and fillna() in Pandas lies in how they deal with lacking values (NaN). dropna() removes rows or columns containing lacking values, successfully decreasing the scale of the DataFrame or Collection. In distinction, fillna() replaces lacking values with specified knowledge, comparable to a hard and fast worth, a computed worth, or by propagating close by values, with out altering the DataFrame’s dimensions. Use dropna() if you wish to exclude incomplete knowledge and fillna() if you wish to retain the dataset’s construction by filling gaps.
Ans. In Pandas, each fillna()
and interpolate()
deal with lacking values however differ in method. fillna()
replaces NaNs with specified values (e.g., constants, imply, median) or propagates present values (e.g., ffill
, bfill
). In distinction, interpolate()
estimates lacking values utilizing surrounding knowledge, making it ultimate for numerical knowledge with logical traits. Primarily, fillna()
applies express replacements, whereas interpolate()
infers values based mostly on knowledge patterns.