Within the age of more and more giant language fashions and complicated neural networks, optimizing mannequin effectivity has develop into paramount. Weight quantization stands out as an important method for decreasing mannequin dimension and enhancing inference velocity with out vital efficiency degradation. This information offers a hands-on method to implementing and understanding weight quantization, utilizing GPT-2 as our sensible instance.
Studying Targets
- Perceive the basics of weight quantization and its significance in mannequin optimization.
- Study the variations between absmax and zero-point quantization strategies.
- Implement weight quantization strategies on GPT-2 utilizing PyTorch.
- Analyze the affect of quantization on reminiscence effectivity, inference velocity, and accuracy.
- Visualize quantized weight distributions utilizing histograms for insights.
- Consider mannequin efficiency post-quantization by means of textual content technology and perplexity metrics.
- Discover some great benefits of quantization for deploying fashions on resource-constrained gadgets.
This text was revealed as part of the Knowledge Science Blogathon.
Understanding Weight Quantization Fundamentals
Weight quantization converts high-precision floating-point weights (sometimes 32-bit) to lower-precision representations (generally 8-bit integers). This course of considerably reduces mannequin dimension and reminiscence utilization whereas making an attempt to protect mannequin efficiency. The important thing problem lies in sustaining mannequin accuracy whereas decreasing numerical precision.
Why Quantize?
- Reminiscence Effectivity: Lowering precision from 32-bit to 8-bit can theoretically scale back mannequin dimension by 75%
- Quicker Inference: Integer operations are usually sooner than floating-point operations
- Decrease Energy Consumption: Decreased reminiscence bandwidth and easier computations result in power financial savings
- Deployment Flexibility: Smaller fashions could be deployed on resource-constrained gadgets
Sensible Implementation
Let’s dive into implementing two common quantization strategies: absmax quantization and zero-point quantization.
Setting Up the Surroundings
First, we’ll arrange our growth setting with essential dependencies:
import seaborn as sns
import torch
import numpy as np
from transformers import AutoModelForCausalLM, AutoTokenizer
from copy import deepcopy
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
import seaborn as sns
Beneath we are going to look into implementing quantization strategies:
Absmax Quantization
The absmax quantization technique scales weights primarily based on the utmost absolute worth within the tensor:
# Outline quantization features
def absmax_quantize(X):
scale = 100 / torch.max(torch.abs(X)) # Adjusted scale
X_quant = (scale * X).spherical()
X_dequant = X_quant / scale
return X_quant.to(torch.int8), X_dequant
This technique works by:
- Discovering the utmost absolute worth within the weight tensor
- Computing a scaling issue to suit values inside int8 vary
- Scaling and rounding the values
- Offering each quantized and dequantized variations
Key benefits:
- Easy implementation
- Good preservation of huge values
- Symmetric quantization round zero
Zero-point Quantization
Zero-point quantization provides an offset to higher deal with uneven distributions:
def zeropoint_quantize(X):
x_range = torch.max(X) - torch.min(X)
x_range = 1 if x_range == 0 else x_range
scale = 200 / x_range
zeropoint = (-scale * torch.min(X) - 128).spherical()
X_quant = torch.clip((X * scale + zeropoint).spherical(), -128, 127)
X_dequant = (X_quant - zeropoint) / scale
return X_quant.to(torch.int8), X_dequant
Output:
Utilizing gadget: cuda
This technique:
- Calculates the complete vary of values
- Determines scale and zero-point parameters
- Applies scaling and shifting
- Clips values to make sure int8 bounds
Advantages:
- Higher dealing with of uneven distributions
- Improved illustration of near-zero values
- Usually ends in higher general accuracy
Loading and Getting ready the Mannequin
Let’s apply these quantization strategies to an actual mannequin. We’ll use GPT-2 as our instance:
# Load mannequin and tokenizer
model_id = 'gpt2'
mannequin = AutoModelForCausalLM.from_pretrained(model_id).to(gadget)
tokenizer = AutoTokenizer.from_pretrained(model_id)
# Print mannequin dimension
print(f"Mannequin dimension: {mannequin.get_memory_footprint():,} bytes")
Output:
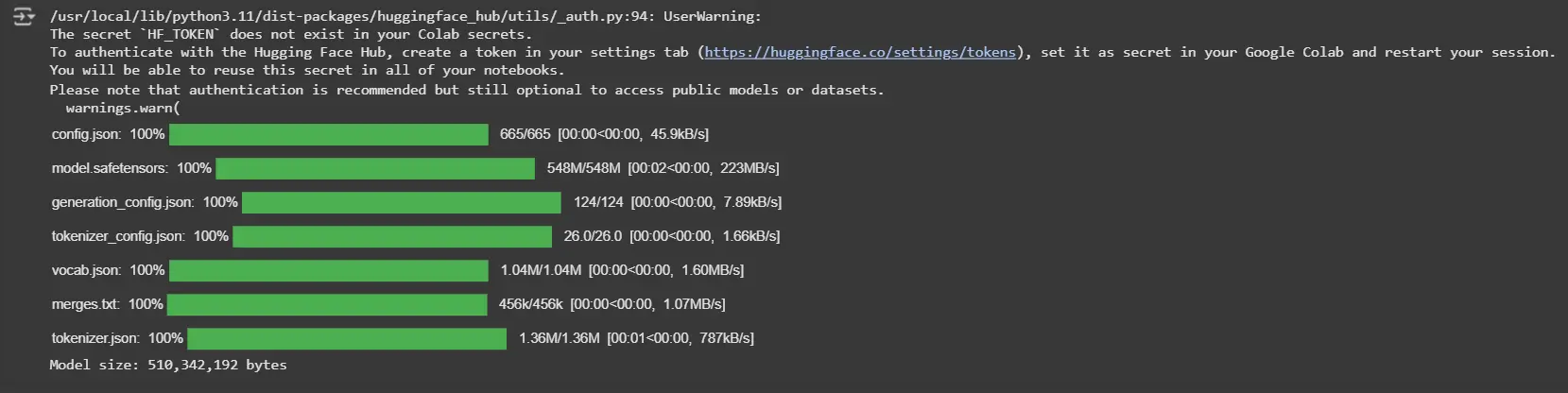
Quantization Course of: Weights and Mannequin
Dive into making use of quantization strategies to each particular person weights and your entire mannequin. This step ensures lowered reminiscence utilization and computational effectivity whereas sustaining efficiency.
# Quantize and visualize weights
weights_abs_quant, _ = absmax_quantize(weights)
weights_zp_quant, _ = zeropoint_quantize(weights)
# Quantize your entire mannequin
model_abs = deepcopy(mannequin)
model_zp = deepcopy(mannequin)
for param in model_abs.parameters():
_, dequantized = absmax_quantize(param.knowledge)
param.knowledge = dequantized
for param in model_zp.parameters():
_, dequantized = zeropoint_quantize(param.knowledge)
param.knowledge = dequantized
Visualizing Quantized Weight Distributions
Visualize and examine the burden distributions of the unique, absmax quantized, and zero-point quantized fashions. These histograms present insights into how quantization impacts weight values and their general distribution.
# Visualize histograms of weights
def visualize_histograms(original_weights, absmax_weights, zp_weights):
sns.set_theme(fashion="darkgrid")
fig, axs = plt.subplots(2, figsize=(10, 10), dpi=300, sharex=True)
axs[0].hist(original_weights, bins=100, alpha=0.6, label="Authentic weights", shade="navy", vary=(-1, 1))
axs[0].hist(absmax_weights, bins=100, alpha=0.6, label="Absmax weights", shade="orange", vary=(-1, 1))
axs[1].hist(original_weights, bins=100, alpha=0.6, label="Authentic weights", shade="navy", vary=(-1, 1))
axs[1].hist(zp_weights, bins=100, alpha=0.6, label="Zero-point weights", shade="inexperienced", vary=(-1, 1))
for ax in axs:
ax.legend()
ax.set_xlabel('Weights')
ax.set_ylabel('Frequency')
ax.yaxis.set_major_formatter(ticker.EngFormatter())
axs[0].set_title('Authentic vs Absmax Quantized Weights')
axs[1].set_title('Authentic vs Zero-point Quantized Weights')
plt.tight_layout()
plt.present()
# Flatten weights for visualization
original_weights = np.concatenate([param.data.cpu().numpy().flatten() for param in model.parameters()])
absmax_weights = np.concatenate([param.data.cpu().numpy().flatten() for param in model_abs.parameters()])
zp_weights = np.concatenate([param.data.cpu().numpy().flatten() for param in model_zp.parameters()])
visualize_histograms(original_weights, absmax_weights, zp_weights)
The code features a complete visualization perform:
- Graph displaying Authentic Weights vs Absmax Weights
- Graph displaying Authentic Weights vs Zero-point Weights
Output:
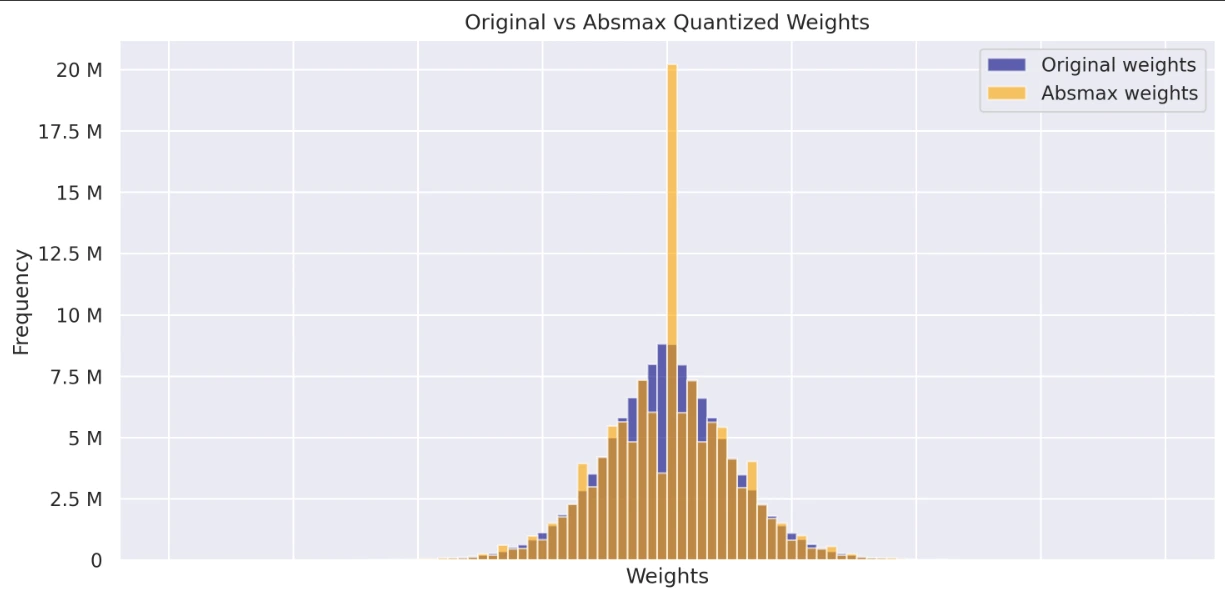
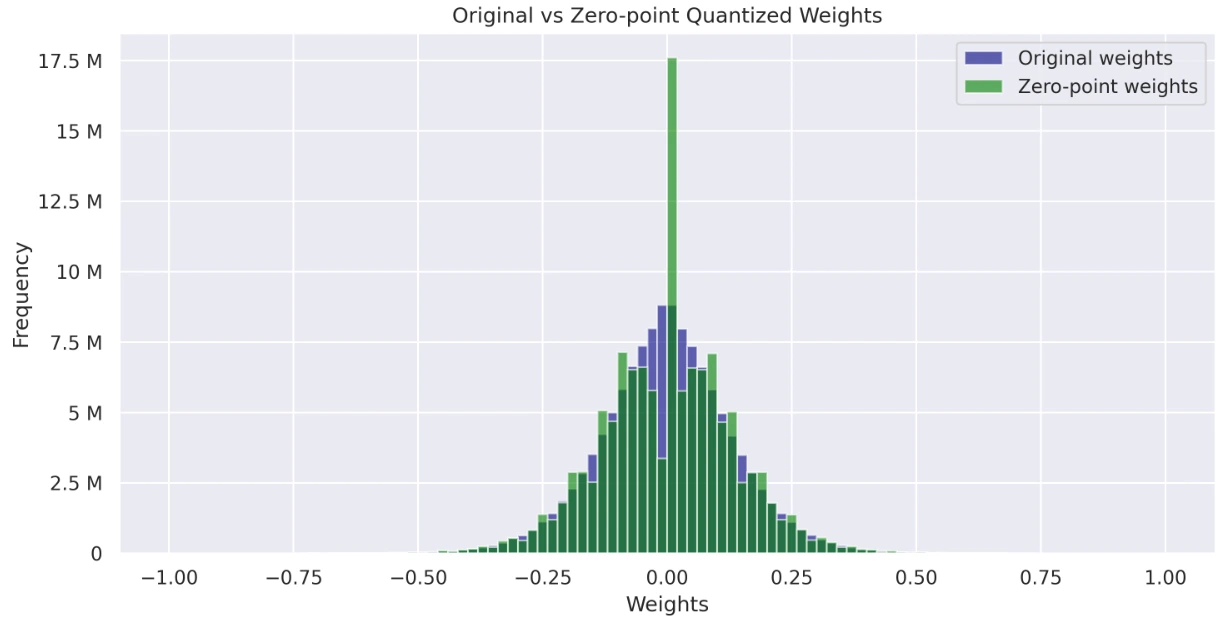
Efficiency Analysis
Evaluating the affect of quantization on mannequin efficiency is important to make sure effectivity and accuracy. Let’s measure how effectively the quantized fashions carry out in comparison with the unique.
Textual content Era
Discover how the quantized fashions generate textual content and examine the standard of outputs to the unique mannequin’s predictions.
def generate_text(mannequin, input_text, max_length=50):
input_ids = tokenizer.encode(input_text, return_tensors="pt").to(gadget)
output = mannequin.generate(inputs=input_ids,
max_length=max_length,
do_sample=True,
top_k=30,
pad_token_id=tokenizer.eos_token_id,
attention_mask=input_ids.new_ones(input_ids.form))
return tokenizer.decode(output[0], skip_special_tokens=True)
# Generate textual content with authentic and quantized fashions
original_text = generate_text(mannequin, "The way forward for AI is")
absmax_text = generate_text(model_abs, "The way forward for AI is")
zp_text = generate_text(model_zp, "The way forward for AI is")
print(f"Authentic mannequin:n{original_text}")
print("-" * 50)
print(f"Absmax mannequin:n{absmax_text}")
print("-" * 50)
print(f"Zeropoint mannequin:n{zp_text}")
This code compares textual content technology outputs from three fashions: the unique, an “absmax” quantized mannequin, and a “zeropoint” quantized mannequin. It makes use of a generate_text perform to generate textual content primarily based on an enter immediate, making use of sampling with a top-k worth of 30. Lastly, it prints the outcomes from all three fashions.
Output:

# Perplexity analysis
def calculate_perplexity(mannequin, textual content):
encodings = tokenizer(textual content, return_tensors="pt").to(gadget)
input_ids = encodings.input_ids
with torch.no_grad():
outputs = mannequin(input_ids, labels=input_ids)
return torch.exp(outputs.loss)
long_text = "Synthetic intelligence is a transformative know-how that's reshaping industries."
ppl_original = calculate_perplexity(mannequin, long_text)
ppl_absmax = calculate_perplexity(model_abs, long_text)
ppl_zp = calculate_perplexity(model_zp, long_text)
print(f"nPerplexity (Authentic): {ppl_original.merchandise():.2f}")
print(f"Perplexity (Absmax): {ppl_absmax.merchandise():.2f}")
print(f"Perplexity (Zero-point): {ppl_zp.merchandise():.2f}")
The code calculates the perplexity (a measure of how effectively a mannequin predicts textual content) for a given enter utilizing three fashions: the unique, “absmax” quantized, and “zeropoint” quantized fashions. Decrease perplexity signifies higher efficiency. It prints the perplexity scores for comparability.
Output:

You’ll be able to entry colab hyperlink right here.
Benefits of Weight Quantization
Beneath we are going to look into some great benefits of weight quantization:
- Reminiscence Effectivity: Quantization reduces mannequin dimension by as much as 75%, enabling sooner loading and inference.
- Quicker Inference: Integer operations are sooner than floating-point operations, resulting in faster mannequin execution.
- Decrease Energy Consumption: Decreased reminiscence bandwidth and simplified computation result in power financial savings, important for edge gadgets and cell deployment.
- Deployment Flexibility: Smaller fashions are simpler to deploy on {hardware} with restricted assets (e.g., cellphones, embedded gadgets).
- Minimal Efficiency Degradation: With the precise quantization technique, fashions can retain most of their accuracy regardless of the lowered precision.
Conclusion
Weight quantization performs an important position in enhancing the effectivity of huge language fashions, significantly in the case of deploying them on resource-constrained gadgets. By changing high-precision weights to lower-precision integer representations, we are able to considerably scale back reminiscence utilization, enhance inference velocity, and decrease energy consumption, all with out severely affecting the mannequin’s efficiency.
On this information, we explored two common quantization strategies—absmax quantization and zero-point quantization—utilizing GPT-2 as a sensible instance. Each strategies demonstrated the flexibility to cut back the mannequin’s reminiscence footprint and computational necessities whereas sustaining a excessive degree of accuracy in textual content technology duties. Nevertheless, the zero-point quantization technique, with its uneven method, usually resulted in higher preservation of mannequin accuracy, particularly for non-symmetric weight distributions.
Key Takeaways
- Absmax Quantization is easier and works effectively for symmetric weight distributions, although it may not seize uneven distributions as successfully as zero-point quantization.
- Zero-point Quantization provides a extra versatile method by introducing an offset to deal with uneven distributions, typically main to higher accuracy and a extra environment friendly illustration of weights.
- Quantization is important for deploying giant fashions in real-time functions the place computational assets are restricted.
- Regardless of the quantization course of decreasing precision, it’s doable to keep up mannequin efficiency near the unique with correct tuning and quantization methods.
- Visualization strategies like histograms can present insights into how quantization impacts mannequin weights and the distribution of values within the tensors.
Regularly Requested Questions
A. Weight quantization reduces the precision of a mannequin’s weights, sometimes from 32-bit floating-point values to lower-precision integers (e.g., 8-bit integers), to avoid wasting reminiscence and computation whereas sustaining efficiency.
A. Whereas quantization reduces the mannequin’s reminiscence footprint and inference time, it could possibly result in a slight degradation in accuracy. Nevertheless, if finished appropriately, the loss in accuracy is minimal.
A. Sure, quantization could be utilized to any neural community mannequin, together with language fashions, imaginative and prescient fashions, and different deep studying architectures.
A. You’ll be able to implement quantization by creating features to scale and around the mannequin’s weights, then apply them throughout all parameters. Libraries like PyTorch present native assist for some quantization strategies, although customized implementations, as proven within the information, provide flexibility.
A. Weight quantization is handiest for big fashions the place decreasing reminiscence footprint and computation is important. Nevertheless, very small fashions might not profit as a lot from quantization.
The media proven on this article just isn’t owned by Analytics Vidhya and is used on the Creator’s discretion.