Andrew Ng just lately launched AISuite, an open-source Python package deal designed to streamline the usage of giant language fashions (LLMs) throughout a number of suppliers. This progressive device simplifies the complexities of working with various LLMs by permitting seamless switching between fashions with a easy “supplier:mannequin” string. By considerably decreasing integration overhead, AISuite enhances flexibility and accelerates software growth, making it a useful useful resource for builders navigating the dynamic panorama of AI. On this article, we are going to see how efficient it’s.
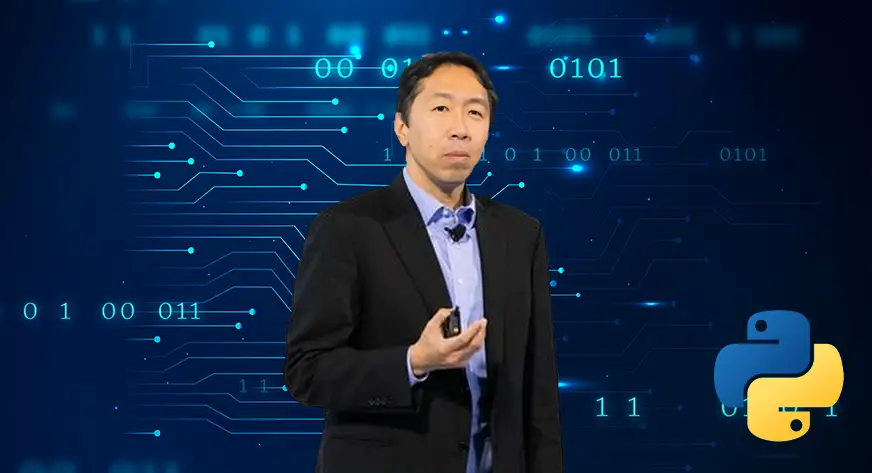
What’s AISuite?
AISuite is an open-source mission led by Andrew Ng, designed to make working with a number of giant language mannequin (LLM) suppliers simpler and extra environment friendly. Obtainable on GitHub, it gives a easy, unified interface that enables seamless switching between LLMs utilizing HTTP endpoints or SDKs, following OpenAI’s interface. This device is good for college students, educators, and builders, providing constant and hassle-free interactions throughout varied suppliers.
Supported by a workforce of open-source contributors, AISuite bridges the hole between completely different LLM frameworks. It permits customers to combine and examine fashions from suppliers like OpenAI, Anthropic, and Meta’s Llama with ease. The device simplifies duties corresponding to producing textual content, conducting analyses, and constructing interactive programs. With options like streamlined API key administration, customizable shopper configurations, and an intuitive setup, AISuite helps each easy purposes and complicated LLM-based tasks.
Implementation of AISuite
1. Set up Essential Libraries
!pip set up openai
!pip set up aisuite[all]
- !pip set up openai: Installs the OpenAI Python library, which is required to work together with OpenAI’s GPT fashions.
- !pip set up aisuite[all]: Installs AISuite together with elective dependencies wanted to help a number of LLM suppliers.
2. Set API Keys for Authentication
os.environ['OPENAI_API_KEY'] = getpass('Enter your OPENAI API key: ')
os.environ['ANTHROPIC_API_KEY'] = getpass('Enter your ANTHROPIC API key: ')
- os.environ: Units setting variables to securely retailer the API keys required to entry LLM providers.
- getpass(): Prompts the consumer to enter their OpenAI and Anthropic API keys securely (with out displaying the enter).
- These keys authenticate your requests to the respective platforms.
Additionally learn: How you can Generate Your Personal OpenAI API Key and Add Credit?
3. Initialize the AISuite Shopper
shopper = ai.Shopper()
This initializes an occasion of the AISuite shopper, permitting interplay with a number of LLMs in a standardized manner.
4. Outline the Immediate (Messages)
messages = [
{"role": "system", "content": "Talk using Pirate English."},
{"role": "user", "content": "Tell a joke in 1 line."}
]
- The messages checklist defines a dialog enter:
- position: “system”: Gives directions to the mannequin (e.g., “Speak utilizing Pirate English”).
- position: “consumer”: Represents the consumer’s question (e.g., “Inform a joke in 1 line”).
- This immediate ensures the responses observe a pirate theme and embrace a one-line joke.
5. Question the OpenAI Mannequin
response = shopper.chat.completions.create(mannequin="openai:gpt-4o", messages=messages, temperature=0.75)
print(response.decisions[0].message.content material)
- mannequin=”openai:gpt-4o”: Specifies the OpenAI GPT-4o mannequin.
- messages=messages: Sends the immediate outlined earlier to the mannequin.
- temperature=0.75: Controls the randomness of the response. A better temperature leads to extra artistic outputs, whereas decrease values produce extra deterministic responses.
- response.decisions[0].message.content material: Extracts the textual content content material of the mannequin’s response.
6. Question the Anthropic Mannequin
response = shopper.chat.completions.create(mannequin="anthropic:claude-3-5-sonnet-20241022", messages=messages, temperature=0.75)
print(response.decisions[0].message.content material)
- mannequin=”anthropic:claude-3-5-sonnet-20241022″: Specifies the Anthropic Claude-3-5 mannequin.
- The remaining parameters are similar to the OpenAI question. This demonstrates how AISuite permits straightforward switching between suppliers by altering the mannequin parameter.
7. Question the Ollama Mannequin
response = shopper.chat.completions.create(mannequin="ollama:llama3.1:8b", messages=messages, temperature=0.75)
print(response.decisions[0].message.content material)
- mannequin=”ollama:llama3.1:8b”: Specifies the Ollama Llama3.1 mannequin.
- Once more, the parameters and logic are constant, showcasing how AISuite gives a unified interface throughout suppliers.
Output
Why did the pirate go to highschool? To enhance his "arrrrrrr-ticulation"!Arrr, why do not pirates take a bathe earlier than they stroll the plank? As a result of
they're going to simply wash up on shore later! 🏴☠️Why did the scurvy canine's parrot go to the physician? As a result of it had a fowl
mood, savvy?
Create a Chat Completion
!pip set up openai
!pip set up aisuite[all]
os.environ['OPENAI_API_KEY'] = getpass('Enter your OPENAI API key: ')
from getpass import getpass
import aisuite as ai
shopper = ai.Shopper()
supplier = "openai"
model_id = "gpt-4o"
messages = [
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "Give me a tabular comparison of RAG and AGENTIC RAG"},
]
response = shopper.chat.completions.create(
mannequin=f"{supplier}:{model_id}",
messages=messages,
)
print(response.decisions[0].message.content material)
Output
Actually! Beneath is a tabular comparability of Retrieval-Augmented Technology
(RAG) and Agentic RAG.| Function | RAG |
Agentic RAG ||------------------------|-------------------------------------------------|-
---------------------------------------------------|| Definition | A framework that mixes retrieval from exterior
paperwork with era. | An extension of RAG that comes with actions
based mostly on exterior interactions and dynamic decision-making. || Parts | - Retrieval System (e.g., a search engine or
doc database) <br> - Generator (e.g., a language mannequin) | - Retrieval
System <br> - Generator <br> - Agentic Layer (action-taking and interplay
controller) || Performance | Retrieves related paperwork and generates
responses based mostly on prompted inputs mixed with the retrieved data.
| Provides the aptitude to take actions based mostly on interactions, corresponding to
interacting with APIs, controlling units, or dynamically gathering extra
data. || Use Circumstances | - Information-based query answering <br> -
Content material summarization <br> - Open-domain dialogue programs | - Autonomous
brokers <br> - Interactive programs <br> - Determination-making purposes <br> -
Programs requiring context-based actions || Interplay | Restricted to the enter retrieval and output
era cycle. | Can work together with exterior programs or interfaces to
collect information, execute duties, and alter the setting based mostly on goal
capabilities. || Complexity | Usually easier because it combines retrieval with
era with out taking actions past producing textual content. | Extra complicated due
to its potential to work together with and modify the state of exterior
environments. || Instance of Utility | Answering complicated questions by retrieving elements of
paperwork and synthesizing them into coherent solutions. | Implementing a
digital assistant able to performing duties like scheduling appointments
by accessing calendars, or a chatbot that manages customer support queries
by means of actions. || Flexibility | Restricted to the out there retrieval corpus and
era mannequin capabilities. | Extra versatile as a result of action-oriented
interactions that may adapt to dynamic environments and circumstances. || Determination-Making Capacity| Restricted decision-making based mostly on static retrieval
and era. | Enhanced decision-making by means of dynamic interplay and
adaptive habits. |This comparability outlines the foundational variations and capabilities
between conventional RAG programs and the extra superior, interaction-capable
Agentic RAG frameworks.
Every mannequin Makes use of a Totally different Supplier
1. Putting in and Importing Libraries
!pip set up aisuite[all]
from pprint import pprint as pp
- Installs the aisuite library with all elective dependencies.
- Imports a pretty-printing perform (pprint) to format output for higher readability. A customized pprint perform is outlined to permit a customized width.
2. Setting Up API Keys
import os
from getpass import getpass
os.environ['GROQ_API_KEY'] = getpass('Enter your GROQ API key: ')
Prompts the consumer to enter their GROQ API key, which is saved within the setting variable GROQ_API_KEY.
3. Initializing the AI Shopper
import aisuite as ai
shopper = ai.Shopper()
Initializes an AI shopper utilizing the aisuite library to work together with completely different fashions.
4. Chat Completions
messages = [
{"role": "system", "content": "You are a helpful agent, who answers with brevity."},
{"role": "user", "content": 'Hi'},
]
response = shopper.chat.completions.create(mannequin="groq:llama-3.2-3b-preview", messages=messages)
print(response.decisions[0].message.content material)
Output
How can I help you?
- Defines a chat with two messages:
- A system message that units the tone or habits of the AI (concise responses).
- A consumer message as enter.
- Sends the messages to the AI mannequin groq:llama-3.2-3b-preview and prints the mannequin’s response.
5. Perform to Ship Queries
def ask(message, sys_message="You're a useful agent.",
mannequin="groq:llama-3.2-3b-preview"):
shopper = ai.Shopper()
messages = [
{"role": "system", "content": sys_message},
{"role": "user", "content": message}
]
response = shopper.chat.completions.create(mannequin=mannequin, messages=messages)
return response.decisions[0].message.content material
ask("Hello. what's capital of Japan?")
Output
'Hiya. The capital of Japan is Tokyo.'
- ask is a reusable perform to ship queries to the mannequin.
- Accepts:
- message: The consumer’s question.
- sys_message: Non-compulsory system instruction.
- mannequin: Specifies the AI mannequin.
- Sends the enter and returns the AI’s response.
6. Utilizing A number of APIs
os.environ['OPENAI_API_KEY'] = getpass('Enter your OPENAI API key: ')
os.environ['ANTHROPIC_API_KEY'] = getpass('Enter your ANTHROPIC API key: ')
print(ask("Who's your creator?"))
print(ask('Who's your creator?', mannequin="anthropic:claude-3-5-sonnet-20240620"))
print(ask('Who's your creator?', mannequin="openai:gpt-4o"))
Output
I used to be created by Meta AI, a number one synthetic intelligence analysis
group. My data was developed from a big corpus of textual content, which
I exploit to generate human-like responses to consumer queries.I used to be created by Anthropic.
I used to be developed by OpenAI, a company that focuses on synthetic
intelligence analysis and deployment.
- Prompts the consumer for OpenAI and Anthropic API keys.
- Sends a question (“Who’s your creator?”) to completely different fashions:
- groq:llama-3.2-3b-preview
- anthropic:claude-3-5-sonnet-20240620
- openai:gpt-4o
- Prints the response from every mannequin, displaying how completely different programs interpret the identical question.
7. Querying A number of Fashions
fashions = [
'llama-3.1-8b-instant',
'llama-3.2-1b-preview',
'llama-3.2-3b-preview',
'llama3-70b-8192',
'llama3-8b-8192'
]
ret = []
for x in fashions:
ret.append(ask('Write a brief one sentence rationalization of the origins of AI?', mannequin=f'groq:{x}'))
- A listing of various mannequin identifiers (fashions) is outlined.
- Loops by means of every mannequin and queries it with:
- Write a brief one sentence rationalization of the origins of AI?
- Shops responses within the checklist ret.
8. Displaying Mannequin Responses
for idx, x in enumerate(ret):
pprint(fashions[idx] + ': n ' + x + ' ')
- Loops by means of the saved responses.
- Codecs and prints the mannequin’s identify together with its response, making it straightforward to match outputs.
Output
('llama-3.1-8b-instant: n'' The origins of Synthetic Intelligence (AI) date again to the 1956 Dartmouth '
'Summer season Analysis Undertaking on Synthetic Intelligence, the place a bunch of '
'laptop scientists, led by John McCarthy, Marvin Minsky, Nathaniel '
'Rochester, and Claude Shannon, coined the time period and laid the inspiration for '
'the event of AI as a definite discipline of examine. ')
('llama-3.2-1b-preview: n'
' The origins of Synthetic Intelligence (AI) date again to the mid-Twentieth '
'century, when the primary laptop applications, which mimicked human-like '
'intelligence by means of algorithms and rule-based programs, had been developed by '
'famend mathematicians and laptop scientists, together with Alan Turing, '
'Marvin Minsky, and John McCarthy within the Nineteen Fifties. ')
('llama-3.2-3b-preview: n'
' The origins of Synthetic Intelligence (AI) date again to the Nineteen Fifties, with '
'the Dartmouth Summer season Analysis Undertaking on Synthetic Intelligence, led by '
'laptop scientists John McCarthy, Marvin Minsky, and Nathaniel Rochester, '
'marking the delivery of AI as a proper discipline of analysis. ')
('llama3-70b-8192: n'
' The origins of Synthetic Intelligence (AI) could be traced again to the Nineteen Fifties '
'when laptop scientist Alan Turing proposed the Turing Check, a way for '
'figuring out whether or not a machine may exhibit clever habits equal '
'to, or indistinguishable from, that of a human. ')
('llama3-8b-8192: n'
' The origins of Synthetic Intelligence (AI) could be traced again to the '
'Nineteen Fifties, when laptop scientists DARPA funded the event of the primary AI '
'applications, such because the Logical Theorist, which aimed to simulate human '
'problem-solving talents and study from expertise. ')
Fashions present assorted responses to the question concerning the origins of AI, reflecting their coaching and reasoning capabilities. As an illustration:
- Some fashions reference the Dartmouth Summer season Analysis Undertaking on AI.
- Others point out Alan Turing or early DARPA-funded AI applications.
Key Options and Takeaways
- Modularity: The script makes use of reusable capabilities (ask) to make querying environment friendly and customisable.
- Multi-Mannequin Interplay: Showcases the power to work together with varied AI programs, together with GROQ, OpenAI, and Anthropic.
- Comparative Evaluation: Facilitates comparability of responses throughout fashions for insights into their strengths and biases.
- Actual-Time Inputs: Helps dynamic enter for API keys, guaranteeing safe integration.
This script is a superb start line for exploring completely different AI mannequin capabilities and understanding their distinctive behaviours.
Conclusion
AISuite is a vital device for anybody navigating the world of huge language fashions. It empowers customers to harness the perfect of a number of AI suppliers whereas simplifying growth and fostering innovation. Its open-source nature and considerate design underscore its potential as a contemporary AI software growth cornerstone.
It accelerates growth and enhances flexibility by enabling seamless switching between fashions like OpenAI, Anthropic, and Meta with minimal integration effort. Perfect for each easy and complicated purposes, AISuite helps modular workflows, API key administration, and real-time multi-model comparisons. Its ease of use, scalability, and skill to streamline cross-provider interactions make it a useful useful resource for builders, researchers, and educators, empowering environment friendly and progressive utilisation of various LLMs in an evolving AI panorama.
In case you are in search of generative AI course on-line then discover: GenAI Pinnacle Program
Ceaselessly Requested Questions
Ans. AISuite is an open-source Python package deal created by Andrew Ng to streamline working with a number of giant language fashions (LLMs) from varied suppliers. It gives a unified interface for switching between fashions, simplifying integration and accelerating growth.
Ans. Sure, AISuite helps querying a number of fashions from completely different suppliers concurrently. You’ll be able to ship the identical question to completely different fashions and examine their responses.
Ans. AISuite’s key function is its modularity and skill to combine a number of LLMs right into a single workflow. It additionally simplifies API key administration and permits straightforward switching between fashions, facilitating fast comparisons and experimentation.
Ans. To put in AISuite and mandatory libraries, run:!pip set up aisuite[all]
!pip set up openai