Within the period of massive knowledge and fast technological development, the power to investigate and interpret knowledge successfully has turn into a cornerstone of decision-making and innovation. Python, famend for its simplicity and flexibility, has emerged because the main programming language for knowledge evaluation. Its in depth library ecosystem permits customers to seamlessly deal with numerous duties, from knowledge manipulation and visualization to superior statistical modeling and machine studying. This text explores the highest 10 Python libraries for knowledge evaluation. Whether or not you’re a newbie or an skilled skilled, these libraries supply scalable and environment friendly options to deal with right now’s knowledge challenges.
1. NumPy
NumPy is the inspiration for numerical computing in Python. This Python library for knowledge evaluation helps massive arrays and matrices and supplies a set of mathematical capabilities for working on these knowledge constructions.
Benefits:
- Effectively handles massive datasets with multidimensional arrays.
- In depth help for mathematical operations like linear algebra and Fourier transforms.
- Integration with different libraries like Pandas and SciPy.
Limitations:
- Lacks high-level knowledge manipulation capabilities.
- Requires Pandas for working with labeled knowledge.
import numpy as np
# Creating an array and performing operations
knowledge = np.array([1, 2, 3, 4, 5])
print("Array:", knowledge)
print("Imply:", np.imply(knowledge))
print("Normal Deviation:", np.std(knowledge))
Output

2. Pandas
Pandas is a knowledge manipulation and evaluation library that introduces DataFrames for tabular knowledge, making it simple to scrub and manipulate structured datasets.
Benefits:
- Simplifies knowledge wrangling and preprocessing.
- Gives high-level capabilities for merging, filtering, and grouping datasets.
- Sturdy integration with NumPy.
Limitations:
- Slower efficiency for terribly massive datasets.
- Consumes vital reminiscence for operations on huge knowledge.
import pandas as pd
# Making a DataFrame
knowledge = pd.DataFrame({'Identify': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'Rating': [85, 90, 95]})
print("DataFrame:n", knowledge)
# Information manipulation
print("Common Age:", knowledge['Age'].imply())
print("Filtered DataFrame:n", knowledge[data['Score'] > 90])
Output
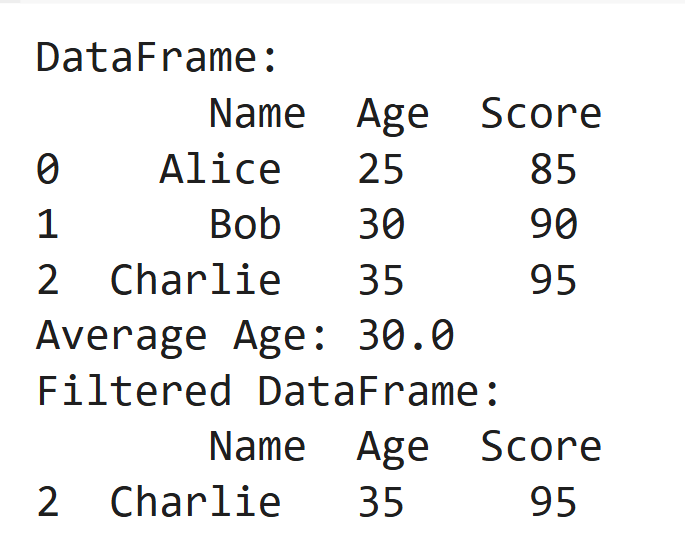
3. Matplotlib
Matplotlib is a plotting library that permits the creation of static, interactive, and animated visualizations.
Benefits:
- Extremely customizable visualizations.
- Serves as the bottom for libraries like Seaborn and Pandas plotting.
- Big selection of plot sorts (line, scatter, bar, and so on.).
Limitations:
- Advanced syntax for superior visualizations.
- Restricted aesthetic attraction in comparison with trendy libraries.
import matplotlib.pyplot as plt
# Information for plotting
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# Plotting
plt.plot(x, y, label="Line Plot")
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Matplotlib Instance')
plt.legend()
plt.present()
Output
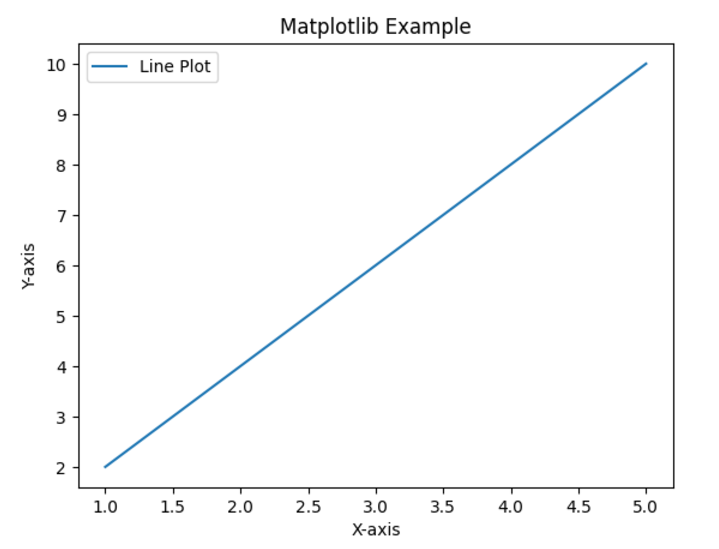
4. Seaborn
Seaborn, Python library for knowledge evaluation, is constructed on Matplotlib and simplifies the creation of statistical visualizations with a deal with enticing aesthetics.
Benefits:
- Straightforward-to-create, aesthetically pleasing plots.
- Constructed-in themes and shade palettes for enhanced visuals.
- Simplifies statistical plots like heatmaps and pair plots.
Limitations:
- Depends on Matplotlib for backend performance.
- Restricted customization in comparison with Matplotlib.
import seaborn as sns
import matplotlib.pyplot as plt
# Pattern knowledge
knowledge = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
# Plotting a histogram
sns.histplot(knowledge, kde=True)
plt.title('Seaborn Histogram')
plt.present()
Output
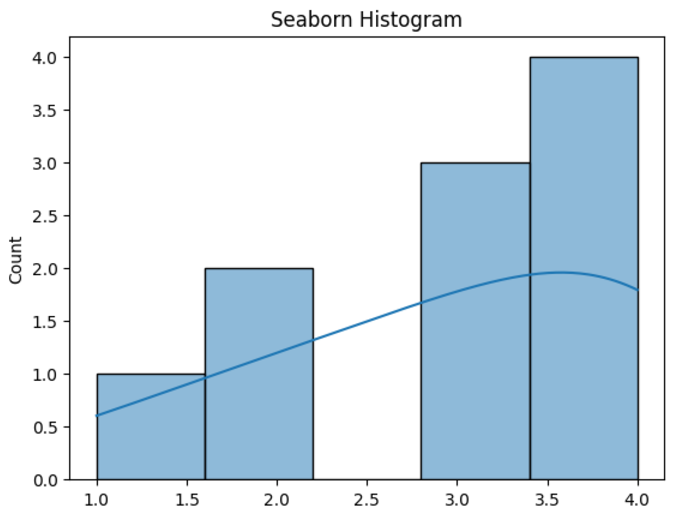
5. SciPy
SciPy builds on NumPy to offer instruments for scientific computing, together with modules for optimization, integration, and sign processing.
Benefits:
- Complete library for scientific duties.
- In depth documentation and examples.
- Integrates nicely with NumPy and Pandas.
Limitations:
- Requires familiarity with scientific computations.
- Not appropriate for high-level knowledge manipulation duties.
from scipy.stats import ttest_ind
# Pattern knowledge
group1 = [1, 2, 3, 4, 5]
group2 = [2, 3, 4, 5, 6]
# T-test
t_stat, p_value = ttest_ind(group1, group2)
print("T-Statistic:", t_stat)
print("P-Worth:", p_value)
Output
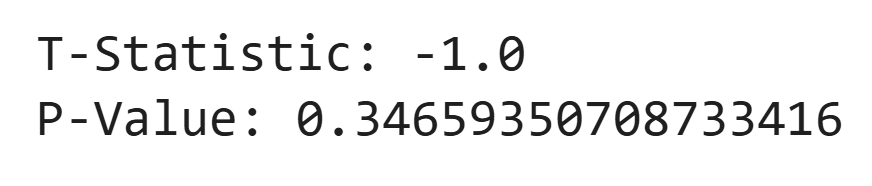
6. Scikit-learn
Scikit-learn is a machine studying library, providing classification, regression, clustering, and extra instruments.
Benefits:
- Consumer-friendly API with well-documented capabilities.
- Huge number of prebuilt machine studying fashions.
- Sturdy integration with Pandas and NumPy.
Limitations:
- Restricted help for deep studying.
- Not designed for large-scale distributed coaching.
from sklearn.linear_model import LinearRegression
# Information
X = [[1], [2], [3], [4]] # Options
y = [2, 4, 6, 8] # Goal
# Mannequin
mannequin = LinearRegression()
mannequin.match(X, y)
print("Prediction for X=5:", mannequin.predict([[5]])[0])
Output

7. Statsmodels
Statsmodels, Python library for knowledge evaluation, supplies instruments for statistical modeling and speculation testing, together with linear fashions and time collection evaluation.
Benefits:
- Superb for econometrics and statistical analysis.
- Detailed output for statistical checks and fashions.
- Sturdy deal with speculation testing.
Limitations:
- Steeper studying curve for learners.
- Slower in comparison with Scikit-learn for predictive modeling.
import statsmodels.api as sm
# Information
X = [1, 2, 3, 4]
y = [2, 4, 6, 8]
X = sm.add_constant(X) # Add fixed for intercept
# Mannequin
mannequin = sm.OLS(y, X).match()
print(mannequin.abstract())
Output
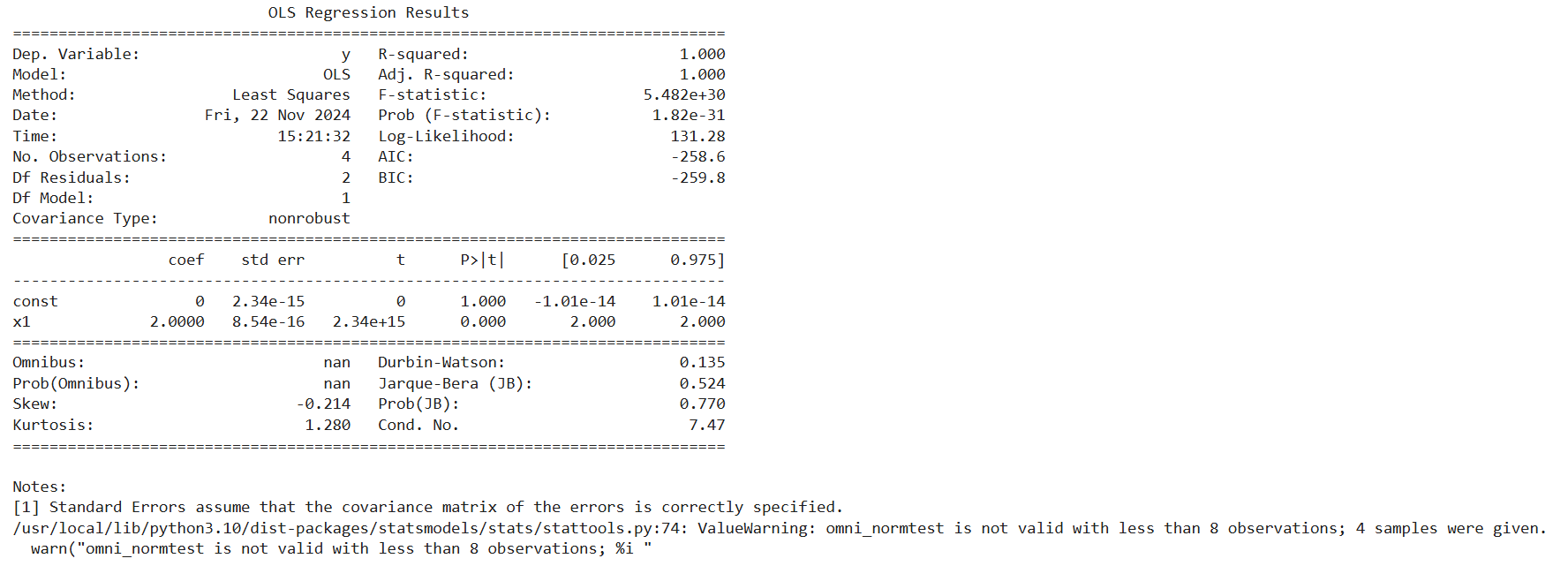
8. Plotly
Plotly is an interactive plotting library used for creating web-based dashboards and visualizations.
Benefits:
- Extremely interactive and responsive visuals.
- Straightforward integration with internet purposes.
- Helps 3D and superior charts.
Limitations:
- Heavier on browser reminiscence for giant datasets.
- Could require further configuration for deployment.
import plotly.specific as px
# Pattern knowledge
knowledge = px.knowledge.iris()
# Scatter plot
fig = px.scatter(knowledge, x="sepal_width", y="sepal_length", shade="species", title="Iris Dataset Scatter Plot")
fig.present()
Output
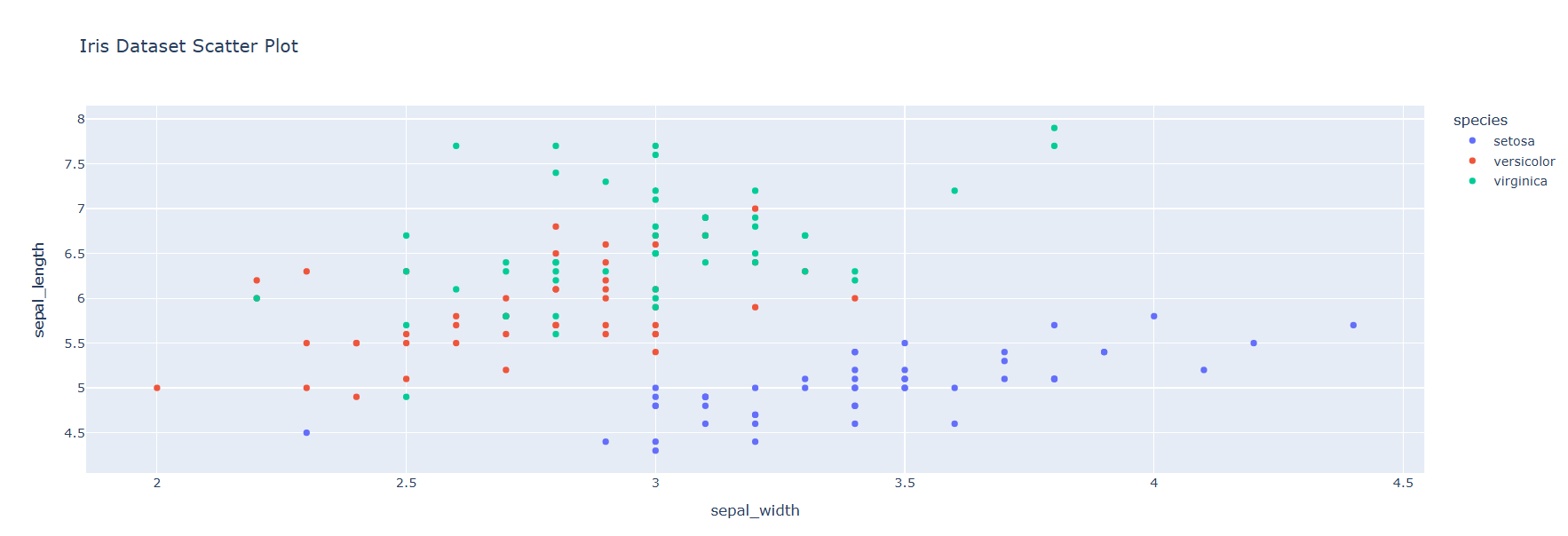
9. PySpark
PySpark is the Python API for Apache Spark, enabling large-scale knowledge processing and distributed computing.
Benefits:
- Handles huge knowledge effectively.
- Integrates nicely with Hadoop and different huge knowledge instruments.
- Helps machine studying with MLlib.
Limitations:
- Requires a Spark setting to run.
- Steeper studying curve for learners.
!pip set up pyspark
from pyspark.sql import SparkSession
# Initialize Spark session
spark = SparkSession.builder.appName("PySpark Instance").getOrCreate()
# Create a DataFrame
knowledge = spark.createDataFrame([(1, "Alice"), (2, "Bob")], ["ID", "Name"])
knowledge.present()
Output

10. Altair
Altair is a declarative statistical visualization library based mostly on Vega and Vega-Lite.
Benefits:
- Easy syntax for creating complicated visualizations.
- Integration with Pandas for seamless knowledge plotting.
Limitations:
- Restricted interactivity in comparison with Plotly.
- Can not deal with extraordinarily massive datasets immediately.
import altair as alt
import pandas as pd
# Easy bar chart
knowledge = pd.DataFrame({'X': ['A', 'B', 'C'], 'Y': [5, 10, 15]})
chart = alt.Chart(knowledge).mark_bar().encode(x='X', y='Y')
chart.show()
Output
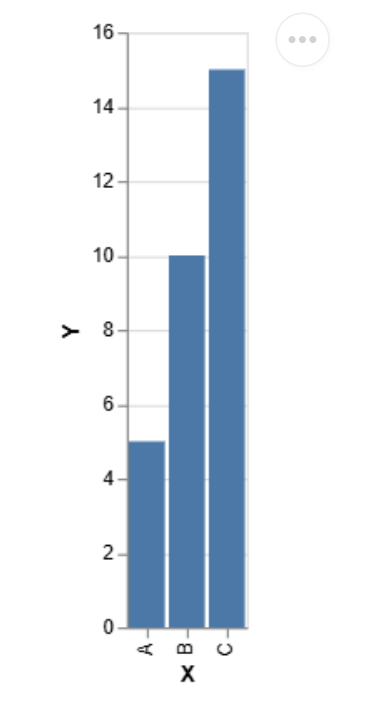
The way to Select the Proper Python Library for Information Evaluation?
Perceive the Nature of Your Job
Step one in choosing a Python library for knowledge evaluation is knowing the precise necessities of your job. Pandas and NumPy are glorious knowledge cleansing and manipulation selections, providing highly effective instruments to deal with structured datasets. Matplotlib supplies primary plotting capabilities for knowledge visualisation, whereas Seaborn creates visually interesting statistical charts. If interactive visualizations are wanted, library like Plotly are splendid. On the subject of statistical evaluation, Statsmodels excels in speculation testing, and SciPy is well-suited for performing superior mathematical operations.
Contemplate Dataset Dimension
The scale of your dataset can affect the selection of libraries. Pandas and NumPy function effectively for small to medium-sized datasets. Nonetheless, when coping with massive datasets or distributed techniques, instruments like PySpark are higher choices. These Python libraries are designed to course of knowledge throughout a number of nodes, making them splendid for large knowledge environments.
Outline Your Evaluation Goals
Your evaluation targets additionally information the library choice. For Exploratory Information Evaluation (EDA), Pandas is a go-to for knowledge inspection, and Seaborn is helpful for producing visible insights. For predictive modeling, Scikit-learn gives an intensive toolkit for preprocessing and implementing machine studying algorithms. In case your focus is on statistical modeling, Statsmodels shines with options like regression evaluation and time collection forecasting.
Prioritize Usability and Studying Curve
Libraries range in usability and complexity. Rookies ought to begin with user-friendly libraries like Pandas and Matplotlib, supported by in depth documentation and examples. Superior customers can discover extra complicated instruments like SciPy, Scikit-learn, and PySpark, that are appropriate for high-level duties however might require a deeper understanding.
Integration and Compatibility
Lastly, make sure the library integrates seamlessly along with your current instruments or platforms. As an illustration, Matplotlib works exceptionally nicely inside Jupyter Notebooks, a well-liked setting for knowledge evaluation. Equally, PySpark is designed for compatibility with Apache Spark, making it splendid for distributed computing duties. Selecting libraries that align along with your workflow will streamline the evaluation course of.
Why Python for Information Evaluation?
Python’s dominance in knowledge evaluation stems from a number of key benefits:
- Ease of Use: Its intuitive syntax lowers the educational curve for newcomers whereas offering superior performance for knowledgeable customers. Python permits analysts to write down clear and concise code, rushing up problem-solving and knowledge exploration.
- In depth Libraries: Python boasts a wealthy library ecosystem designed for knowledge manipulation, statistical evaluation, and visualization.
- Group Assist: Python’s huge, energetic neighborhood contributes steady updates, tutorials, and options, making certain sturdy help for customers in any respect ranges.
- Integration with Large Information Instruments: Python seamlessly integrates with huge knowledge applied sciences like Hadoop, Spark, and AWS, making it a best choice for dealing with massive datasets in distributed techniques.
Conclusion
Python’s huge and numerous library ecosystem makes it a powerhouse for knowledge evaluation, able to addressing duties starting from knowledge cleansing and transformation to superior statistical modeling and visualization. Whether or not you’re a newbie exploring foundational libraries like NumPy, Pandas, and Matplotlib, or a sophisticated consumer leveraging the capabilities of Scikit-learn, PySpark, or Plotly, Python gives instruments tailor-made to each stage of the info workflow.
Selecting the best library hinges on understanding your job, dataset dimension, and evaluation targets whereas contemplating usability and integration along with your current setting. With Python, the chances for extracting actionable insights from knowledge are almost limitless, solidifying its standing as a necessary software in right now’s data-driven world.