Think about having an AI assistant who doesn’t simply reply to your queries however thinks by way of issues systematically, learns from previous experiences, and plans a number of steps earlier than taking motion. Language Agent Tree Search (LATS) is a sophisticated AI framework that mixes the systematic reasoning of ReAct prompting with the strategic planning capabilities of Monte Carlo Tree Search.
LATS operates by sustaining a complete resolution tree, exploring a number of attainable options concurrently, and studying from every interplay to make more and more higher choices. With Vertical AI Brokers being the main target, on this article, we are going to talk about and implement how you can make the most of LATS Brokers in motion utilizing LlamaIndex and SambaNova.AI.Â
Studying Aims
- Perceive the working movement of ReAct (Reasoning + Performing) prompting framework and its thought-action-observation cycle implementation.
- As soon as we perceive the ReAct workflow, we are able to discover the developments made on this framework, notably within the type of the LATS Agent.
- Study to implement the Language Agent Tree Search (LATS) framework primarily based on Monte Carlo Tree Search with language mannequin capabilities.
- Discover the trade-offs between computational sources and final result optimization in LATS implementation to grasp when it’s useful to make use of and when it’s not.
- Implement a Suggestion Engine utilizing LATS Agent from LlamaIndex utilizing SambaNova System as an LLM supplier.
This text was revealed as part of the Knowledge Science Blogathon.
What’s React Agent?

ReAct (Reasoning + Performing) is a prompting framework that allows language fashions to unravel duties by way of a thought, motion, and statement cycle. Consider it like having an assistant who thinks out loud, takes motion, and learns from what they observe. The agent follows a sample:
- Thought: Causes concerning the present scenario
- Motion: Decides what to do primarily based on that reasoning
- Remark: Will get suggestions from the setting
- Repeat: Use this suggestions to tell the following thought
When applied, it allows language fashions to interrupt down issues into manageable elements, make choices primarily based on accessible info, and alter their method primarily based on suggestions. As an example, when fixing a multi-step math downside, the mannequin would possibly first take into consideration which mathematical ideas apply, then take motion by making use of a selected formulation, observe whether or not the end result makes logical sense, and alter its method if wanted. This structured cycle of reasoning and motion carefully behaves as human problem-solving processes and results in extra dependable responses.
Earlier Learn:Â Implementation of ReAct Agent utilizing LlamaIndex and Gemini
What’s a Language Agent Tree Search Agent?
The Language Agent Tree Search (LATS) is a sophisticated Agentic framework that mixes the Monte Carlo Tree Search with language mannequin capabilities to create a greater refined decision-making system for reasoning and planning.

It operates by way of a steady cycle of exploration, analysis, and studying, beginning with an enter question that initiates a structured search course of. The system maintains a complete long-term reminiscence containing each a search tree of earlier explorations and reflections from previous makes an attempt, which helps information future decision-making.
At its operational core, LATS follows a scientific workflow the place it first selects nodes primarily based on promising paths after which samples a number of attainable actions at every resolution level. Every potential motion undergoes a worth perform analysis to evaluate its benefit, adopted by a simulation to a terminal state to find out its effectiveness.Â
Within the code demo, we are going to see how this Tree enlargement works and the way the analysis rating can be executed.Â
How do LATS use REACT?
LATS integrates ReAct’s thought-action-observation cycle into its tree search framework. Right here’s how:
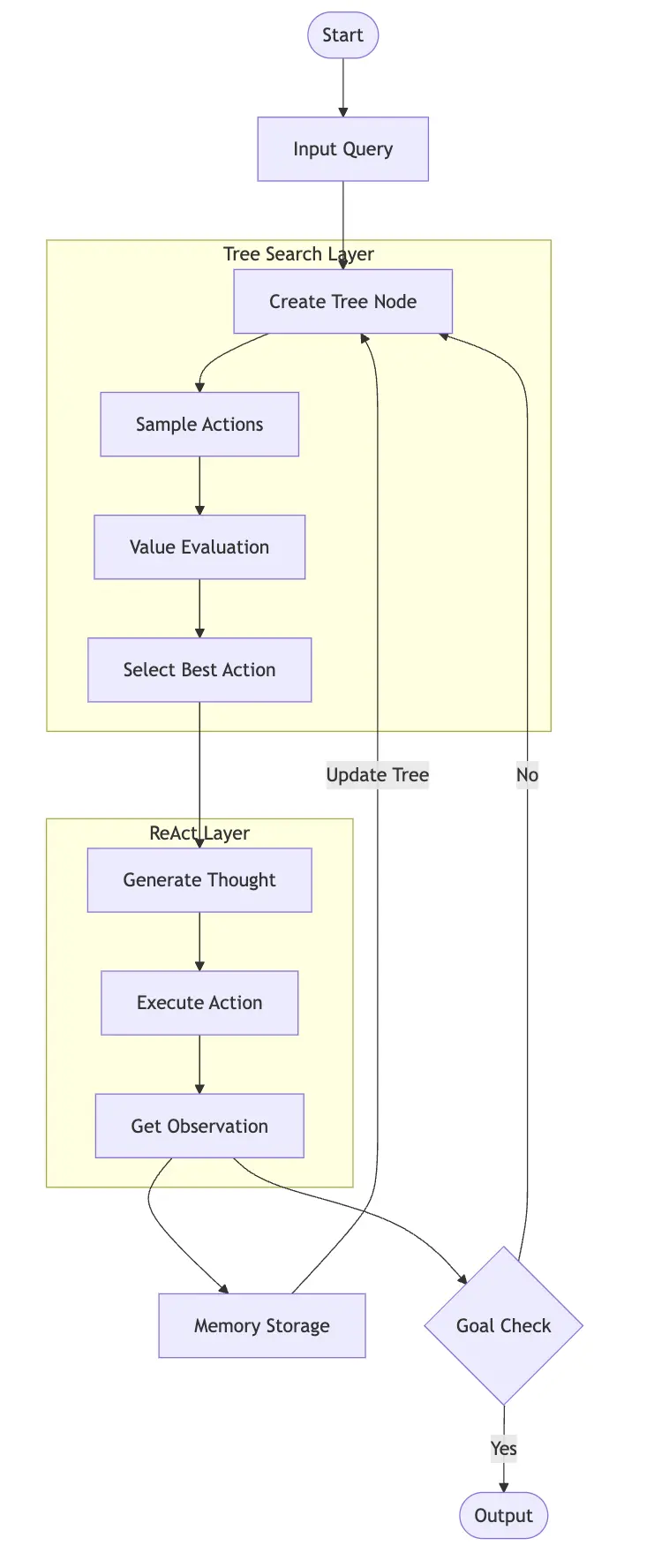
At every node within the search tree, LATS makes use of ReAct’s:
- Thought era to cause concerning the state.
- Motion choice to decide on what to do.
- Remark assortment to get suggestions.
However LATS enhances this by:
- Exploring a number of attainable ReAct sequences concurrently in Tree enlargement i.e., completely different nodes to suppose, and take motion.
- Utilizing previous experiences to information which paths to discover Studying from successes and failures systematically.
This method to implement could be very costly. Let’s perceive when and when to not use LATS.Â
Value Commerce-Offs: When to Use LATS?
Whereas the paper focuses on the upper benchmarks of LATS in comparison with COT, ReAcT, and different methods, the execution comes with the next price. The deeper the complicated duties get, the extra nodes are created for reasoning and planning, which suggests we are going to find yourself making a number of LLM calls – a setup that’s not excellent in manufacturing environments.
This computational depth turns into notably difficult when coping with real-time functions the place response time is crucial, as every node enlargement and analysis requires separate API calls to the language mannequin. Moreover, organizations must fastidiously weigh the trade-off between LATS’s superior decision-making capabilities and the related infrastructure prices, particularly when scaling throughout a number of concurrent customers or functions.
Right here’s when to make use of LATS:
- The duty is complicated and has a number of attainable options (e.g., Programming duties the place there are a lot of methods to unravel an issue).
- Errors are expensive and accuracy is essential (e.g., Monetary decision-making or medical prognosis help, Schooling curriculum preparation).
- The duty advantages from studying from previous makes an attempt (e.g.Advanced product searches the place person preferences matter)
Right here’s when to not use LATS:
- Easy, simple duties that want fast responses (e.g., fundamental customer support inquiries or information lookups)Â
- Time-sensitive operations the place quick choices are required (e.g., real-time buying and selling programs or emergency response)
- Useful resource-constrained environments with restricted computational energy or API finances (e.g., cell functions or edge units)Â
- Excessive-volume, repetitive duties the place less complicated fashions can present sufficient outcomes (e.g., content material moderation or spam detection)
Nonetheless, for easy, simple duties the place fast responses are wanted, the less complicated ReAct framework may be extra acceptable.
Consider it this manner: ReAct is like making choices one step at a time, whereas LATS is like planning a posh technique recreation – it takes extra time and sources however can result in higher outcomes in complicated conditions.
Construct a Suggestion System with LATS Agent utilizing LlamaIndex
Should you’re seeking to construct a advice system that thinks and analyzes the web, let’s break down this implementation utilizing LATS (Language Agent Job System) and LlamaIndex.
Step 1: Setting Up Your Atmosphere
First up, we have to get our instruments so as. Run these pip set up instructions to get all the pieces we want:
!pip set up llama-index-agent-lats
!pip set up llama-index-core llama-index-readers-file
!pip set up duckduckgo-search
!pip set up llama-index-llms-sambanovasystems
import nest_asyncio
nest_asyncio.apply()
Together with nest_asyncio for dealing with async operations in your notebooks.
Step 2: Configuration and API Setup
Right here’s the place we arrange our LLM – the SambaNova LLM. You’ll must create your API key and plug it contained in the setting variable i.e., SAMBANOVA_API_KEY.Â
Observe these steps to get your API key:
- Create your account at: https://cloud.sambanova.ai/
- Choose APIs and select the mannequin it’s good to use.
- You too can click on on the Generate New API key and use that key to switch the beneath setting variable.
import os
os.environ["SAMBANOVA_API_KEY"] = "<replace-with-your-key>"
SambaNova Cloud is taken into account to have the World’s Quickest AI Inference, the place you will get the response from Llama open-source fashions inside seconds. When you outline the LLM from the LlamaIndex LLM integrations, it’s good to override the default LLM utilizing Settings from LlamaIndex core. By default, Llamaindex makes use of OpenAI because the LLM.Â
from llama_index.core import Settings
from llama_index.llms.sambanovasystems import SambaNovaCloud
llm = SambaNovaCloud(
mannequin="Meta-Llama-3.1-70B-Instruct",
context_window=100000,
max_tokens=1024,
temperature=0.7,
top_k=1,
top_p=0.01,
)
Settings.llm = llm
Step 3: Defining Device-Search
Now for the enjoyable half – we’re integrating DuckDuckGo search to assist our system discover related info. This software fetches real-world information for the given person query and fetches the max outcomes of 4.Â
To outline the software i.e., Operate calling within the LLMs all the time bear in mind these two steps:
- Correctly outline the information kind the perform will return, in our case it’s: -> str.
- At all times embody docstrings on your perform name that must be added in Agentic Workflow or Operate calling. Since perform calling can assist in question routing, the Agent must know when to decide on which software to motion, that is the place docstrings are very useful.
Now use FunctionTool from LlamaIndex and outline your customized perform.Â
from duckduckgo_search import DDGS
from llama_index.core.instruments import FunctionTool
def search(question:str) -> str:
"""
Use this perform to get outcomes from Net Search by way of DuckDuckGo
Args:
question: person immediate
return:
context (str): search outcomes to the person question
"""
# def search(question:str)
req = DDGS()
response = req.textual content(question,max_results=4)
context = ""
for end in response:
context += end result['body']
return context
search_tool = FunctionTool.from_defaults(fn=search)
Step 4: LlamaIndex Agent Runner – LATS
That is the ultimate a part of the Agent definition. We have to outline LATSAgent Employee from the LlamaIndex agent. Since it is a Employee class, we additional can run it by way of AgentRunner the place we immediately make the most of the chat perform.
Observe: The chat and different options may also be immediately referred to as from AgentWorker, however it’s higher to make use of AgentRunner, because it has been up to date with a lot of the newest modifications accomplished within the framework.Â
Key hyperparameters:
- num_expansions: Variety of youngsters nodes to increase.
- max_rollouts: Most variety of trajectories to pattern.
from llama_index.agent.lats import LATSAgentWorker
from llama_index.core.agent import AgentRunner
agent_worker = LATSAgentWorker(
instruments=[search_tool],
llm=llm,
num_expansions=2,
verbose=True,
max_rollouts=3)
agent = AgentRunner(agent_worker)
Step 5: Execute Agent
Lastly, it’s time to execute the LATS agent, simply ask the advice it’s good to ask. Throughout the execution, observe the verbose logs:
- LATS Agent divides the person job into num_expansion.
- When it divides this job, it runs the thought course of after which makes use of the related motion to choose the software. In our case, it’s only one software.
- As soon as it runs the rollout and will get the statement, it evaluates the outcomes it generates.
- It repeats this course of and creates a tree node to get one of the best statement attainable.
question = "On the lookout for a mirrorless digital camera below $2000 with good low-light efficiency"
response = agent.chat(question)
print(response.response)
Output:
Listed below are the highest 5 mirrorless cameras below $2000 with good low-light efficiency:1. Nikon Zf – Contains a 240M full-frame BSI ONOS sensor, full-width 4K/30 video, cropped 4K/80, and stabilization rated to SEV.
2. Sony ZfC II – A compact, full-frame mirrorless digital camera with limitless 4K recording, even in low-light circumstances.
3. Fujijiita N-Yu – Presents an ABC-C format, 25.9M X-frame ONOS 4 sensor, and a large native sensitivity vary of ISO 160-12800 for higher efficiency.
4. Panasonic Lunix OHS – A ten Megapixel digital camera with a four-thirds ONOS sensor, able to limitless 4K recording even in low mild.
5. Canon EOS R6 – Geared up with a 280M full-frame ONOS sensor, 4K/60 video, stabilization rated to SEV, and improved low-light efficiency.
Observe: The rating could differ primarily based on particular person preferences and particular wants.
The above method works effectively, however you have to be ready to deal with edge instances. Generally, if the person’s job question is extremely complicated or entails a number of num_expansion or rollouts, there’s a excessive likelihood the output shall be one thing like, “I’m nonetheless pondering.” Clearly, this response isn’t acceptable. In such instances, there’s a hacky method you’ll be able to strive.
Step 6: Error Dealing with and Hacky Approaches
For the reason that LATS Agent creates a node, every node generates a toddler tree. For every little one tree, the Agent retrieves observations. To examine this, it’s good to test the checklist of duties the Agent is executing. This may be accomplished through the use of agent.list_tasks(). The perform will return a dictionary containing the state, from which you’ll determine the root_node and navigate to the final statement to investigate the reasoning executed by the Agent.
print(agent.list_tasks()[0])
print(agent.list_tasks()[0].extra_state.keys())
print(agent.list_tasks()[-1].extra_state["root_node"].youngsters[0].youngsters[0].current_reasoning[-1].statement)
Now everytime you get I’m nonetheless pondering simply use this hack method to get the end result of the end result.Â
def process_recommendation(question: str, agent: AgentRunner):
"""Course of the advice question with error dealing with"""
strive:
response = agent.chat(question).response
if "I'm nonetheless pondering." in response:
return agent.list_tasks()[-1].extra_state["root_node"].youngsters[0].youngsters[0].current_reasoning[-1].statement
else:
return response
besides Exception as e:
return f"An error occurred whereas processing your request: {str(e)}"
Conclusion
Language Agent Tree Search (LATS) represents a big development in AI agent architectures, combining the systematic exploration of Monte Carlo Tree Search with the reasoning capabilities of huge language fashions. Whereas LATS provides superior decision-making capabilities in comparison with less complicated approaches like Chain-of-Thought (CoT) or fundamental ReAct brokers, it comes with elevated computational overhead and complexity.Â
Key Takeaways
- Understood the ReAcT Agent, the approach that’s utilized in a lot of the Agentic frameworks for job execution.
- Analysis on Language Tree Search (LATS), the development for ReAcT agent that makes use of Monte Carlo Tree searches to additional enhance the output response for complicated duties.
- LATS Agent is just excellent for complicated, high-stakes situations requiring accuracy and studying the place latency isn’t a problem.
- Implementation of LATS Agent utilizing a customized search software to get real-world responses for the given person job.
- As a result of complexity of LATS, error dealing with, and probably “hacky” approaches may be wanted to extract ends in sure situations.
Steadily Requested Questions
A. Â LATS enhances ReAct by exploring a number of attainable sequences of ideas, actions, and observations concurrently inside a tree construction, utilizing previous experiences to information the search and studying from successes and failures systematically utilizing analysis the place LLM acts as a Decide.Â
A. Commonplace language fashions primarily deal with producing textual content primarily based on a given immediate. Brokers, like ReAct, go a step additional by with the ability to work together with their setting, take actions primarily based on reasoning, and observe the outcomes to enhance future actions.
A. When establishing a LATS agent in LlamaIndex, key hyperparameters to contemplate embody num_expansions, the breadth of the search by figuring out what number of little one nodes are explored from every level, and max_rollouts, the depth of the search by limiting the variety of simulated motion sequences. Moreover, max_iterations is one other optionally available parameter that limits the general reasoning cycles of the agent, stopping it from operating indefinitely and managing computational sources successfully.
A. The official implementation for “Language Agent Tree Search Unifies Reasoning, Performing, and Planning in Language Fashions” is obtainable on GitHub: https://github.com/lapisrocks/LanguageAgentTreeSearchÂ
The media proven on this article isn’t owned by Analytics Vidhya and is used on the Writer’s discretion.