Python has grow to be the lingua franca for scientific computing and knowledge visualization, thanks largely to its wealthy ecosystem of libraries. One such software that has been a favorite amongst researchers and practitioners alike is PyLab. On this article, we’ll delve into the world of PyLab, exploring its origins, options, sensible use circumstances, and why it stays a pretty possibility for these working in knowledge science. By the top of this information, you’ll have a deep understanding of PyLab’s capabilities, together with hands-on code examples that illustrate its energy and ease of use.
In knowledge science, the flexibility to quickly prototype, analyze, and visualize knowledge is paramount. Python’s ecosystem affords quite a lot of libraries that simplify these duties. It’s one such library that mixes the capabilities of Matplotlib and NumPy right into a single namespace, permitting customers to carry out numerical operations and create compelling visualizations seamlessly.
This text is structured to offer each theoretical insights and sensible examples. Whether or not you’re a seasoned knowledge scientist or a newbie desperate to discover knowledge visualization, the excellent protection under will provide help to perceive the advantages and limitations of utilizing PyLab in your initiatives.
Studying Targets
- Perceive PyLab – Study what PyLab is and the way it integrates Matplotlib and NumPy.
- Discover Key Options – Establish PyLab’s unified namespace, interactive instruments, and plotting capabilities.
- Apply Knowledge Visualization – Use PyLab to create varied plots for scientific and exploratory evaluation.
- Consider Strengths and Weaknesses – Analyze PyLab’s advantages and limitations in knowledge science initiatives.
- Evaluate Alternate options – Differentiate PyLab from different visualization instruments like Matplotlib, Seaborn, and Plotly.
This text was printed as part of the Knowledge Science Blogathon.
What’s PyLab?
PyLab is a module inside the Matplotlib library that provides a handy MATLAB-like interface for plotting and numerical computation. Primarily, it merges features from each Matplotlib (for plotting) and NumPy (for numerical operations) into one namespace. This integration allows customers to write down concise code for each computing and visualization with out having to import a number of modules individually.
The Twin Nature of PyLab
- Visualization: PyLab consists of quite a lot of plotting features corresponding to plot(), scatter(), hist(), and lots of extra. These features help you create high-quality static, animated, and interactive visualizations.
- Numerical Computing: With built-in help from NumPy, PyLab affords environment friendly numerical operations on giant arrays and matrices. Capabilities corresponding to linspace(), sin(), cos(), and different mathematical operations are available.
A Easy Instance of PyLab
Contemplate the next code snippet that demonstrates the facility of PyLab for making a easy sine wave plot:
# Importing all features from PyLab
from pylab import *
# Generate an array of 100 equally spaced values between 0 and a pair of*pi
t = linspace(0, 2 * pi, 100)
# Compute the sine of every worth within the array
s = sin(t)
# Create a plot with time on the x-axis and amplitude on the y-axis
plot(t, s, label="Sine Wave")
# Add title and labels
title('Sine Wave Visualization')
xlabel('Time (radians)')
ylabel('Amplitude')
legend()
# Show the plot
present()
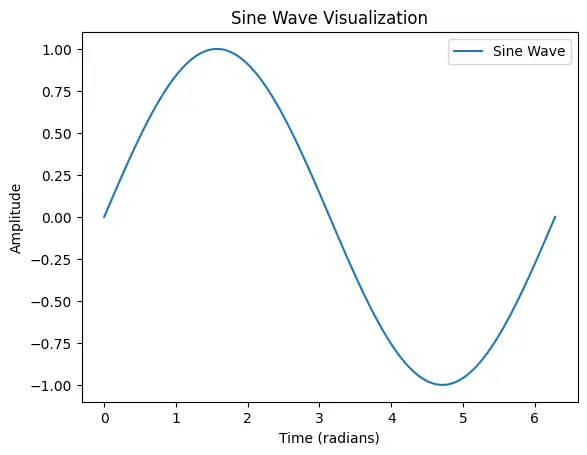
On this instance, features like linspace, sin, and plot are all out there underneath the PyLab namespace, making the code each concise and intuitive.
Key Options of PyLab
PyLab’s integration of numerical and graphical libraries affords a number of noteworthy options:
1. Unified Namespace
One among PyLab’s major options is its capability to deliver collectively quite a few features right into a single namespace. This reduces the necessity to change contexts between totally different libraries. For instance, as an alternative of writing:
# Importing Libraries Explicitly
import numpy as np
import matplotlib.pyplot as plt
t = np.linspace(0, 2*np.pi, 100)
s = np.sin(t)
plt.plot(t, s)
plt.present()
You may merely write:
from pylab import *
t = linspace(0, 2*pi, 100)
s = sin(t)
plot(t, s)
present()
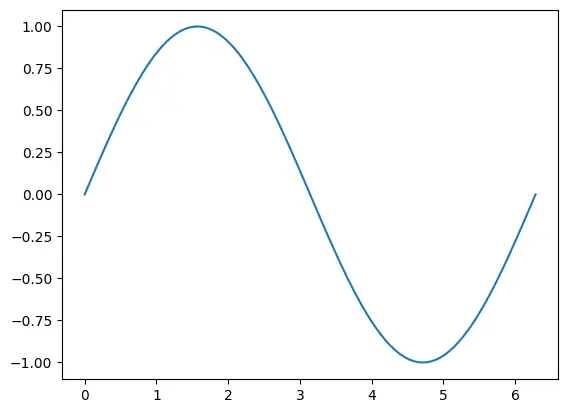
This unified method makes the code simpler to learn and write, notably for fast experiments or interactive evaluation.
2. Interactive Surroundings
PyLab is very efficient in interactive environments corresponding to IPython or Jupyter Notebooks. Its interactive plotting capabilities enable customers to visualise knowledge rapidly and modify plots in real-time. This interactivity is essential for exploratory knowledge evaluation the place fast suggestions loops can drive deeper insights.
3. MATLAB-like Syntax
For customers transitioning from MATLAB, PyLab’s syntax is acquainted and straightforward to undertake. Capabilities like plot(), xlabel(), and title() work equally to their MATLAB counterparts, easing the educational curve for brand spanking new Python customers.
For instance under is the MATLAB code to plot a sine wave :
% Generate an array of 100 values between 0 and a pair of*pi
x = linspace(0, 2*pi, 100);
% Compute the sine of every worth
y = sin(x);
% Create a plot with a crimson strong line of width 2
plot(x, y, 'r-', 'LineWidth', 2);
% Add title and axis labels
title('Sine Wave');
xlabel('Angle (radians)');
ylabel('Sine Worth');
% Allow grid on the plot
grid on;
Whereas that is the PyLab Python code to plot the identical :Â
from pylab import *
# Generate an array of 100 values between 0 and a pair of*pi
x = linspace(0, 2*pi, 100)
# Compute the sine of every worth
y = sin(x)
# Create a plot with a crimson strong line of width 2
plot(x, y, 'r-', linewidth=2)
# Add title and axis labels
title('Sine Wave')
xlabel('Angle (radians)')
ylabel('Sine Worth')
# Allow grid on the plot
grid(True)
# Show the plot
present()
4. Complete Plotting Choices
PyLab helps quite a lot of plot sorts together with:
- Line Plots: Very best for time-series knowledge.
- Scatter Plots: Helpful for visualizing relationships between variables.
- Histograms: Important for understanding knowledge distributions.
- Bar Charts: Excellent for categorical knowledge visualization.
- 3D Plots: For extra advanced knowledge visualization duties.
5. Ease of Customization
PyLab supplies intensive customization choices. You may modify plot aesthetics corresponding to colours, line kinds, markers, and fonts whereas utilizing easy instructions. For instance:
# Import
from pylab import *
# Pattern knowledge
x = linspace(0, 10, 100)
y = exp(-x / 3) * cos(2 * pi * x)
# Create a plot with customized kinds
plot(x, y, 'r--', linewidth=2, marker="o", markersize=5)
title('Damped Oscillation')
xlabel('Time')
ylabel('Amplitude')
grid(True)
present()
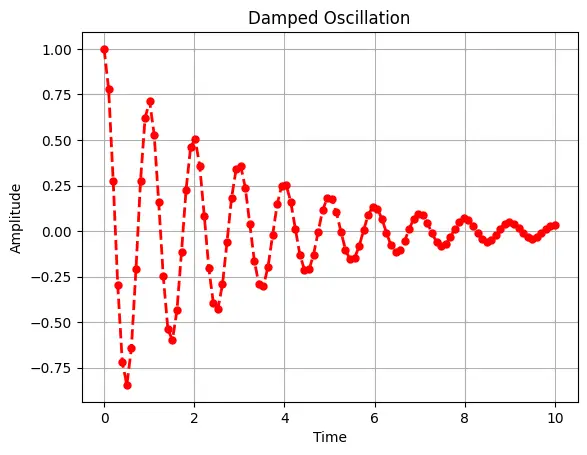
6. Integration with Scientific Libraries
On account of its basis on NumPy and Matplotlib, PyLab integrates easily with different scientific libraries corresponding to SciPy and Pandas. This enables for extra superior statistical evaluation and knowledge manipulation alongside visualization.
# Import Libraries
import pandas as pd
from scipy.optimize import curve_fit
from pylab import *
# Outline the mannequin perform (an exponential decay mannequin)
def model_func(x, A, B):
return A * exp(-B * x)
# Generate artificial knowledge
x_data = linspace(0, 4, 50)
true_params = [2.5, 1.3]
y_clean = model_func(x_data, *true_params)
noise = 0.2 * regular(measurement=x_data.measurement)
y_data = y_clean + noise
# Create a Pandas DataFrame for the information
df = pd.DataFrame({'x': x_data, 'y': y_data})
print("Knowledge Preview:")
print(df.head())
# Use SciPy's curve_fit to estimate the parameters A and B
popt, pcov = curve_fit(model_func, df['x'], df['y'])
print("nFitted Parameters:")
print("A =", popt[0], "B =", popt[1])
# Generate predictions from the fitted mannequin
x_fit = linspace(0, 4, 100)
y_fit = model_func(x_fit, *popt)
# Plot the unique knowledge and the fitted curve utilizing PyLab
determine(figsize=(8, 4))
scatter(df['x'], df['y'], label="Knowledge", shade="blue")
plot(x_fit, y_fit, 'r-', label="Fitted Curve", linewidth=2)
title('Curve Becoming Instance: Exponential Decay')
xlabel('X')
ylabel('Y')
legend()
grid(True)
present()
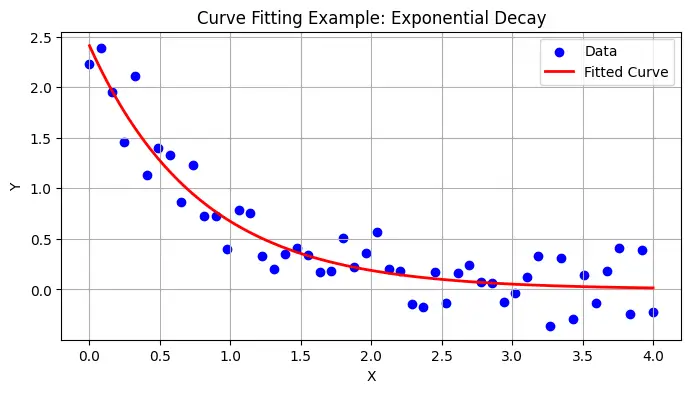
Use Circumstances of PyLab
PyLab’s versatility makes it relevant throughout a variety of scientific and engineering domains. Beneath are some widespread use circumstances the place PyLab excels.
1. Knowledge Visualization in Exploratory Knowledge Evaluation (EDA)
When performing EDA, it’s essential to visualise knowledge to establish tendencies, outliers, and patterns. PyLab’s concise syntax and interactive plotting capabilities make it an ideal software for this function.
Instance: Visualizing a Gaussian Distribution
from pylab import *
# Generate random knowledge following a Gaussian distribution
knowledge = randn(1000)
# Plot a histogram of the information
hist(knowledge, bins=30, shade="skyblue", edgecolor="black")
title('Histogram of Gaussian Distribution')
xlabel('Worth')
ylabel('Frequency')
present()
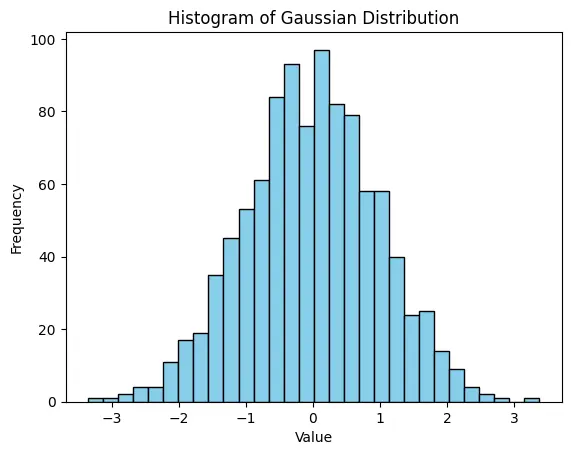
2. Scientific Simulations and Modeling
Researchers typically require fast visualization of simulation outcomes. PyLab can be utilized to plot the evolution of bodily techniques over time, corresponding to oscillatory behaviour in mechanical techniques or wave propagation in physics.
Instance: Damped Oscillator Simulation
from pylab import *
# Time array
t = linspace(0, 10, 200)
# Parameters for a damped oscillator
A = 1.0 # Preliminary amplitude
b = 0.3 # Damping issue
omega = 2 * pi # Angular frequency
# Damped oscillation perform
y = A * exp(-b * t) * cos(omega * t)
# Plot the damped oscillation
plot(t, y, 'b-', linewidth=2)
title('Damped Oscillator Simulation')
xlabel('Time (s)')
ylabel('Amplitude')
grid(True)
present()
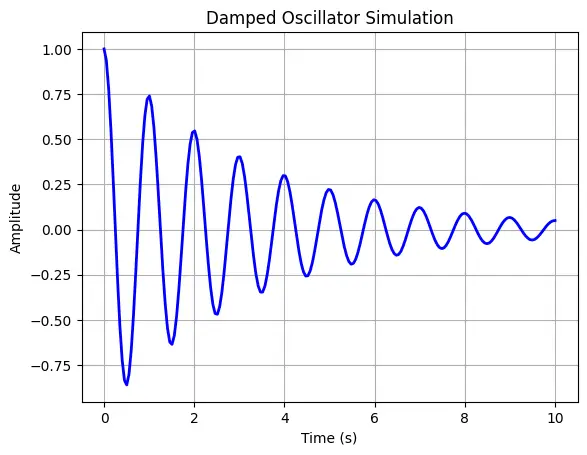
3. Actual-Time Knowledge Monitoring
For functions corresponding to sensor knowledge acquisition or monetary market evaluation, real-time plotting is crucial. PyLab’s interactive mode can be utilized along side reside knowledge streams to replace visualizations on the fly.
Instance: Actual-Time Plotting (Simulated)
from pylab import *
import time
# Allow interactive mode
ion()
# Create a determine and axis
determine()
t_vals = []
y_vals = []
# Simulate real-time knowledge acquisition
for i in vary(100):
t_vals.append(i)
y_vals.append(sin(i * 0.1) + random.randn() * 0.1)
# Clear present determine
clf()
# Plot the up to date knowledge
plot(t_vals, y_vals, 'g-', marker="o")
title('Actual-Time Knowledge Monitoring')
xlabel('Time (s)')
ylabel('Sensor Worth')
# Pause briefly to replace the plot
pause(0.1)
# Flip off interactive mode and show closing plot
ioff()
present()
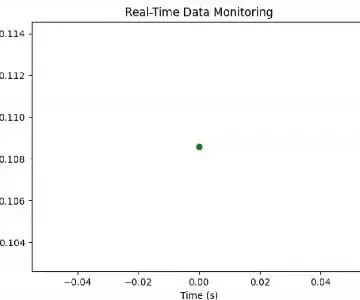
4. Academic Functions and Fast Prototyping
Educators and college students profit enormously from PyLab’s simplicity. Its MATLAB-like interface permits fast demonstration of ideas in arithmetic, physics, and engineering with out intensive boilerplate code. Moreover, researchers can use PyLab for fast prototyping earlier than transitioning to extra advanced manufacturing techniques.
Why You Ought to Use PyLab
Whereas trendy Python programming typically encourages express imports (e.g., importing solely the required features from NumPy or Matplotlib), there are compelling causes to proceed utilizing PyLab in sure contexts:
1. Conciseness and Productiveness
The one-namespace method supplied by PyLab permits for very concise code. That is notably helpful when the first purpose is fast prototyping or interactive exploration of information. As a substitute of juggling a number of imports and namespaces, you’ll be able to focus instantly on the evaluation at hand.
2. Ease of Transition from MATLAB
For scientists and engineers coming from a MATLAB background, PyLab affords a well-recognized atmosphere. The features and plotting instructions mirror MATLAB’s syntax, thereby decreasing the educational curve and facilitating a smoother transition to Python.
3. Interactive Knowledge Exploration
In environments like IPython and Jupyter Notebooks, PyLab’s capability to rapidly generate plots and replace them interactively is invaluable. This interactivity fosters a extra participating evaluation course of, enabling you to experiment with parameters and instantly see the outcomes.
4. Complete Performance
The mixture of Matplotlib’s sturdy plotting capabilities and NumPy’s environment friendly numerical computations in a single module makes PyLab a flexible software. Whether or not you’re visualizing statistical knowledge, working simulations, or monitoring real-time sensor inputs, it supplies the mandatory instruments with out the overhead of managing a number of libraries.
5. Streamlined Studying Expertise
For newbies, having a unified set of features to be taught might be much less overwhelming in comparison with juggling a number of libraries with differing syntax and conventions. This may speed up the educational course of and encourage experimentation.
Conclusion
In conclusion, PyLab supplies an accessible entry level for each newcomers and skilled practitioners in search of to make the most of the facility of Python for scientific computing and knowledge visualization. By understanding its options, exploring its sensible functions, and acknowledging its limitations, you may make knowledgeable choices about when and tips on how to incorporate PyLab into your knowledge science workflow.
PyLab simplifies scientific computing and visualization in Python, offering a MATLAB-like expertise with seamless integration with NumPy, SciPy, and Pandas. Its interactive plotting and intuitive syntax make it best for fast knowledge exploration and prototyping.
Nevertheless, it has some drawbacks. It imports features into the worldwide namespace, which might result in conflicts and is basically deprecated in favour of express Matplotlib utilization. It additionally lacks the pliability of Matplotlib’s object-oriented method and isn’t suited to large-scale functions.
Whereas it’s wonderful for newbies and fast evaluation, transitioning to Matplotlib’s customary API is really useful for extra superior and scalable visualization wants.
Key Takeaways
- Perceive the Fundamentals of PyLab: Study what’s it and the way it integrates Matplotlib and NumPy right into a single namespace for numerical computing and knowledge visualization.
- Discover the Key Options of PyLab: Establish and make the most of its core functionalities, corresponding to its unified namespace, interactive atmosphere, MATLAB-like syntax, and complete plotting choices.
- Apply PyLab for Knowledge Visualization and Scientific Computing: Develop hands-on expertise by creating several types of visualizations, corresponding to line plots, scatter plots, histograms, and real-time knowledge monitoring graphs.
- Consider the Advantages and Limitations of Utilizing PyLab: Analyze the benefits, corresponding to ease of use and fast prototyping, whereas additionally recognizing its drawbacks, together with namespace conflicts and restricted scalability for big functions.
- Evaluate PyLab with Different Approaches: Perceive the variations between PyLab and express Matplotlib/Numpy imports, and discover when to make use of versus different libraries like Seaborn or Plotly for knowledge visualization.
The media proven on this article isn’t owned by Analytics Vidhya and is used on the Writer’s discretion.
Often Requested Questions
Ans. PyLab is a module inside the Matplotlib library that mixes plotting features and numerical operations by importing each Matplotlib and NumPy right into a single namespace. It supplies a MATLAB-like interface, which simplifies plotting and numerical computation in Python.
Ans. Whereas PyLab is superb for interactive work and fast prototyping, many specialists advocate utilizing express imports (e.g., import numpy as np and import matplotlib.pyplot as plt) for manufacturing code. This observe helps keep away from namespace collisions and makes the code extra readable and maintainable.
Ans. Matplotlib is a complete library for creating static, interactive, and animated visualizations in Python. PyLab is basically a comfort module inside Matplotlib that mixes its performance with NumPy’s numerical capabilities right into a single namespace, offering a extra streamlined (and MATLAB-like) interface.
Ans. Completely! PyLab is especially efficient in interactive environments corresponding to IPython and Jupyter Notebooks. Its capability to replace plots in real-time makes it an important software for exploratory knowledge evaluation and academic demonstrations.
Ans. Alternate options embrace utilizing express imports from NumPy and Matplotlib, and even higher-level libraries corresponding to Seaborn for statistical knowledge visualization and Plotly for interactive web-based plots. These alternate options supply extra management over the code and might be higher suited to advanced or large-scale initiatives.