OpenAI’s newest mannequin, o3-mini, is revolutionizing coding duties with its superior reasoning, problem-solving, and code era capabilities. It effectively handles advanced queries and integrates structured knowledge, setting a brand new normal in AI purposes. This text explores utilizing o3-mini and CrewAI to construct a Retrieval-Augmented Era (RAG) analysis assistant agent that retrieves info from a number of PDFs and processes person queries intelligently. We are going to use CrewAI’s CrewDoclingSource, SerperDevTool, and OpenAI’s o3-mini to boost automation in analysis workflows.
Constructing the RAG Agent with o3-mini and CrewAI
With the overwhelming quantity of analysis being printed, an automatic RAG-based assistant might help researchers shortly discover related insights with out manually skimming by a whole bunch of papers. The agent we’re going to construct will course of PDFs to extract key info and reply queries primarily based on the content material of the paperwork. If the required info isn’t discovered within the PDFs, it’s going to routinely carry out an internet search to offer related insights. This setup may be prolonged for extra superior duties, comparable to summarizing a number of papers, detecting contradictory findings, or producing structured experiences.
On this hands-on information, we’ll construct a analysis agent that can undergo articles on DeepSeek-R1 and o3-mini, to reply queries we ask about these fashions. For constructing this analysis assistant agent, we’ll first undergo the conditions and arrange the atmosphere. We are going to then import the mandatory modules, set the API keys, and cargo the analysis paperwork. Then, we’ll go on to outline the AI mannequin and combine the online search device into it. Lastly, we’ll create he AI brokers, outline their duties, and assemble the crew. As soon as prepared, we’ll run the analysis assistant agent to seek out out if o3-mini is best and safer than DeepSeek-R1.
Conditions
Earlier than diving into the implementation, let’s briefly go over what we have to get began. Having the best setup ensures a easy improvement course of and avoids pointless interruptions.
So, guarantee you might have:
- A working Python atmosphere (3.8 or above)
- API keys for OpenAI and Serper (Google Scholar API)
With these in place, we’re prepared to begin constructing!
Step 1: Set up Required Libraries
First, we have to set up the mandatory libraries. These libraries present the muse for the doc processing, AI agent orchestration, and internet search functionalities.
!pip set up crewai
!pip set up 'crewai[tools]'
!pip set up docling
These libraries play a vital position in constructing an environment friendly AI-powered analysis assistant.
- CrewAI gives a strong framework for designing and managing AI brokers, permitting the definition of specialised roles and enabling environment friendly analysis automation. It additionally facilitates process delegation, guaranteeing easy collaboration between AI brokers.
- Moreover, CrewAI[tools] installs important instruments that improve AI brokers’ capabilities, enabling them to work together with APIs, carry out internet searches, and course of knowledge seamlessly.
- Docling makes a speciality of extracting structured data from analysis paperwork, making it superb for processing PDFs, educational papers, and text-based information. On this undertaking, it’s used to extract key findings from arXiv analysis papers.
Step 2: Import Essential Modules
import os
from crewai import LLM, Agent, Crew, Job
from crewai_tools import SerperDevTool
from crewai.data.supply.crew_docling_source import CrewDoclingSource
On this,
- The os module securely manages environmental variables like API keys for easy integration.
- LLM powers the AI reasoning and response era.
- The Agent defines specialised roles to deal with duties effectively.
- Crew manages a number of brokers, guaranteeing seamless collaboration.
- Job assigns and tracks particular obligations.
- SerperDevTool permits Google Scholar searches, bettering exterior reference retrieval.
- CrewDoclingSource integrates analysis paperwork, enabling structured data extraction and evaluation.
Step 3: Set API Keys
os.environ['OPENAI_API_KEY'] = 'your_openai_api_key'
os.environ['SERPER_API_KEY'] = 'your_serper_api_key'
The right way to Get API Keys?
- OpenAI API Key: Join at OpenAI and get an API key.
- Serper API Key: Register at Serper.dev to acquire an API key.
These API keys permit entry to AI fashions and internet search capabilities.
Step 4: Load Analysis Paperwork
On this step, we’ll load the analysis papers from arXiv, enabling our AI mannequin to extract insights from them. The chosen papers cowl key matters:
- https://arxiv.org/pdf/2501.12948: Explores incentivizing reasoning capabilities in LLMs by reinforcement studying (DeepSeek-R1).
- https://arxiv.org/pdf/2501.18438: Compares the protection of o3-mini and DeepSeek-R1.
- https://arxiv.org/pdf/2401.02954: Discusses scaling open-source language fashions with a long-term perspective.
content_source = CrewDoclingSource(
file_paths=[
"https://arxiv.org/pdf/2501.12948",
"https://arxiv.org/pdf/2501.18438",
"https://arxiv.org/pdf/2401.02954"
],
)
Step 5: Outline the AI Mannequin
Now we’ll outline the AI mannequin.
llm = LLM(mannequin="o3-mini", temperature=0)
- o3-mini: A robust AI mannequin for reasoning.
- temperature=0: Ensures deterministic outputs (similar reply for a similar question).
Step 6: Configure Internet Search Software
To reinforce analysis capabilities, we combine an internet search device that retrieves related educational papers when the required info will not be discovered within the supplied paperwork.
serper_tool = SerperDevTool(
search_url="https://google.serper.dev/scholar",
n_results=2 # Fetch high 2 outcomes
)
- search_url=”https://google.serper.dev/scholar”
This specifies the Google Scholar search API endpoint.It ensures that searches are carried out particularly in scholarly articles, analysis papers, and educational sources, relatively than normal internet pages.
- n_results=2
This parameter limits the variety of search outcomes returned by the device, guaranteeing that solely essentially the most related info is retrieved. On this case, it’s set to fetch the highest two analysis papers from Google Scholar, prioritizing high-quality educational sources. By decreasing the variety of outcomes, the assistant retains responses concise and environment friendly, avoiding pointless info overload whereas sustaining accuracy.
Step 7: Outline Embedding Mannequin for Doc Search
To effectively retrieve related info from paperwork, we use an embedding mannequin that converts textual content into numerical representations for similarity-based search.
embedder = {
"supplier": "openai",
"config": {
"mannequin": "text-embedding-ada-002",
"api_key": os.environ['OPENAI_API_KEY']
}
}
The embedder in CrewAI is used for changing textual content into numerical representations (embeddings), enabling environment friendly doc retrieval and semantic search. On this case, the embedding mannequin is supplied by OpenAI, particularly utilizing “text-embedding-ada-002”, a well-optimized mannequin for producing high-quality embeddings. The API secret’s retrieved from the atmosphere variables to authenticate requests.
CrewAI helps a number of embedding suppliers, together with OpenAI and Gemini (Google’s AI fashions), permitting flexibility in selecting the very best mannequin primarily based on accuracy, efficiency, and price concerns.
Step 8: Create the AI Brokers
Now we’ll create the 2 AI Brokers required for our researching process: the Doc Search Agent, and the Internet Search Agent.
The Doc Search Agent is accountable for retrieving solutions from the supplied analysis papers and paperwork. It acts as an knowledgeable in analyzing technical content material and extracting related insights. If the required info will not be discovered, it might probably delegate the question to the Internet Search Agent for additional exploration. The allow_delegation=True setting permits this delegation course of.
doc_agent = Agent(
position="Doc Searcher",
purpose="Discover solutions utilizing supplied paperwork. If unavailable, delegate to the Search Agent.",
backstory="You're an knowledgeable in analyzing analysis papers and technical blogs to extract insights.",
verbose=True,
allow_delegation=True, # Permits delegation to the search agent
llm=llm,
)
The Internet Search Agent, however, is designed to seek for lacking info on-line utilizing Google Scholar. It steps in solely when the Doc Search Agent fails to seek out a solution within the accessible paperwork. In contrast to the Doc Search Agent, it can not delegate duties additional (allow_delegation=False). It makes use of Serper (Google Scholar API) as a device to fetch related educational papers and guarantee correct responses.
search_agent = Agent(
position="Internet Searcher",
purpose="Seek for the lacking info on-line utilizing Google Scholar.",
backstory="When the analysis assistant can not discover a solution, you step in to fetch related knowledge from the online.",
verbose=True,
allow_delegation=False,
instruments=[serper_tool],
llm=llm,
)
Step 9: Outline the Duties for the Brokers
Now we’ll create the 2 duties for the brokers.
The primary process includes answering a given query utilizing accessible analysis papers and paperwork.
task1 = Job(
description="Reply the next query utilizing the accessible paperwork: {query}. "
"If the reply will not be discovered, delegate the duty to the Internet Search Agent.",
expected_output="A well-researched reply from the supplied paperwork.",
agent=doc_agent,
)
The subsequent process comes into play when the document-based search doesn’t yield a solution.
Job 2: Carry out Internet Search if Wanted
task2 = Job(
description="If the document-based agent fails to seek out the reply, carry out an internet search utilizing Google Scholar.",
expected_output="An online-searched reply with related citations.",
agent=search_agent,
)
Step 10: Assemble the Crew
The Crew in CrewAI manages brokers to finish duties effectively by coordinating the Doc Search Agent and Internet Search Agent. It first searches throughout the uploaded paperwork and delegates to internet search if wanted.
- knowledge_sources=[content_source] gives related paperwork,
- embedder=embedder permits semantic search, and
- verbose=True logs actions for higher monitoring, guaranteeing a easy workflow.
crew = Crew(
brokers=[doc_agent, search_agent],
duties=[task1, task2],
verbose=True,
knowledge_sources=[content_source],
embedder=embedder
)
Step 11: Run the Analysis Assistant
The preliminary question is directed to the doc to verify if the researcher agent can present a response. The query being requested is “O3-MINI vs DEEPSEEK-R1: Which one is safer?”
Instance Question 1:
query = "O3-MINI VS DEEPSEEK-R1: WHICH ONE IS SAFER?"
outcome = crew.kickoff(inputs={"query": query})
print("Remaining Reply:n", outcome)
Response:
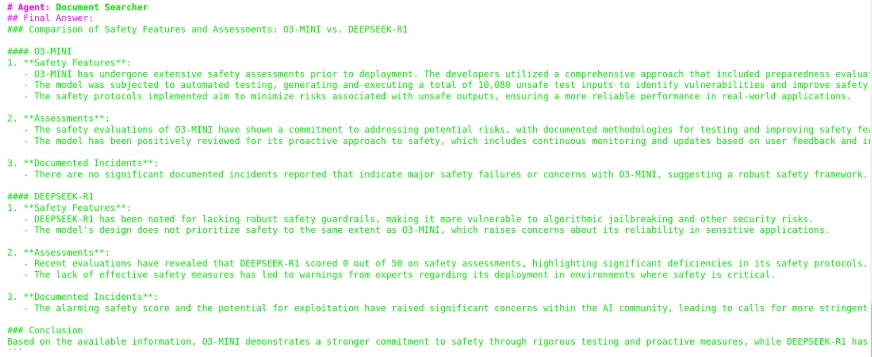
Right here, we are able to see that the ultimate reply is generated by the Doc Searcher, because it efficiently positioned the required info throughout the supplied paperwork.
Instance Question 2:
Right here, the query “Which one is best, O3 Mini or DeepSeek R1?” will not be accessible within the doc. The system will verify if the Doc Search Agent can discover a solution; if not, it’s going to delegate the duty to the Internet Search Agent
query = "Which one is best O3 Mini or DeepSeek R1?"
outcome = crew.kickoff(inputs={"query": query})
print("Remaining Reply:n", outcome)
Response:
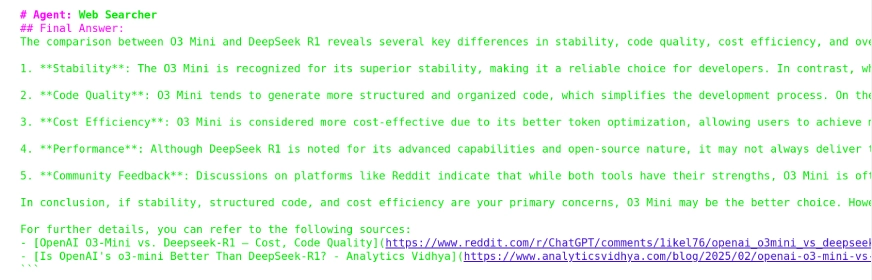
From the output, we observe that the response was generated utilizing the Internet Searcher Agent for the reason that required info was not discovered by the Doc Researcher Agent. Moreover, it consists of the sources from which the reply was lastly retrieved.
Conclusion
On this undertaking, we efficiently constructed an AI-powered analysis assistant that effectively retrieves and analyzes info from analysis papers and the online. Through the use of CrewAI for agent coordination, Docling for doc processing, and Serper for scholarly search, we created a system able to answering advanced queries with structured insights.
The assistant first searches inside paperwork and seamlessly delegates to internet search if wanted, guaranteeing correct responses. This strategy enhances analysis effectivity by automating info retrieval and evaluation. Moreover, by integrating the o3-mini analysis assistant with CrewAI’s CrewDoclingSource and SerperDevTool, we additional enhanced the system’s doc evaluation capabilities. With additional customization, this framework may be expanded to assist extra knowledge sources, superior reasoning, and improved analysis workflows.
You possibly can discover wonderful initiatives that includes OpenAI o3-mini in our free course – Getting Began with o3-mini!
Steadily Requested Questions
A. CrewAI is a framework that lets you create and handle AI brokers with particular roles and duties. It permits collaboration between a number of AI brokers to automate advanced workflows.
A. CrewAI makes use of a structured strategy the place every agent has an outlined position and may delegate duties if wanted. A Crew object orchestrates these brokers to finish duties effectively.
A. CrewDoclingSource is a doc processing device in CrewAI that extracts structured data from analysis papers, PDFs, and text-based paperwork.
A. Serper API is a device that enables AI purposes to carry out Google Search queries, together with searches on Google Scholar for educational papers.
A. Serper API provides each free and paid plans, with limitations on the variety of search requests within the free tier.
A. In contrast to normal Google Search, Serper API gives structured entry to look outcomes, permitting AI brokers to extract related analysis papers effectively.
A. Sure, it helps frequent analysis doc codecs, together with PDFs and text-based information.